Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial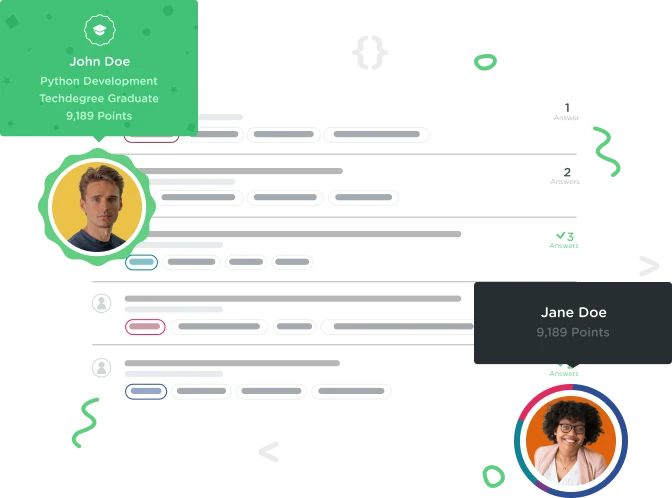
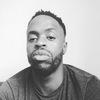
olu adesina
23,007 PointsC# is there a way to convert string to an operator (+/*-) and how do i get the number1 variable into scope?
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp3
{
class Program
{
static void Main(string[] args)
{
while (true)
{
Console.Write("Enter a random number: ");
string input1=Console.ReadLine();
if (input1.ToLower() =="quit")
{
break;
}
try
{
int number1 = int.Parse(input1);
Console.Write("Enter a random +,/,- or *: ");//convert this to an operator
string math = Console.ReadLine();
Console.Write("Enter another random number: ");
string input2 = Console.ReadLine();
if (input2.ToLower() == "quit")
{
break;
}
try
{
int number2 = int.Parse(input2);
Console.WriteLine(input1 + math + input2 + "=" number1 + number2); // how can i get number one in scope
}
catch (FormatException)
{
Console.WriteLine("wrong input numbers only");
continue;
}
}
catch (FormatException)
{
Console.WriteLine("wrong input numbers only");
continue;
}
}
}
}
}
2 Answers

Tom Kapon
5,360 PointsHi,
when you declare variables inside of a class or the main method you should allways declare them at the beginning of the class or main method so they have a global scope to the main method or class the only time you declare variables elsewhere than the beginning of your class or main method is when you want to create local variables inside of a specific method so that it can only be used inside this method. for the string conversion to an operator you can't convert the symbols but you could use generics to convert strings to operations in the following way.
public static Func<T, T, bool> ConvertToBinaryConditionOperator<T>(string op) { switch (op) { case "<": return Operator.LessThan<T>; case ">": return Operator.GreaterThan<T>; case "==": return Operator.Equal<T>; case "<=": return Operator.LessThanOrEqual<T>; // etc default: throw new ArgumentException("op"); } }

James Churchill
Treehouse TeacherOlu,
To convert a string to an operator in your app, I would keep it simple and create a method like the following:
private int PerformCalculation(string operator, int number1, int number2)
{
if (operator == "+")
{
return number1 + number2;
}
else if (operator == "-")
{
return number1 - number2;
}
else if (operator == "*")
{
return number1 * number2;
}
else if (operator == "/")
{
// Warning: Integer division probably won't produce the result you're looking for.
// Try using `double` instead of `int` for your numbers.
return number1 / number2;
}
else
{
throw new ArgumentException("Unexpected operator string: " + operator);
}
}
And you'd call it like this:
int result = PerformCalculation("+", 1, 2);
Also, the number1
variable appears to be in scope to me. What is the error message that you're receiving from the compiler? What I do see is a missing "+" operator in this line of code:
Console.WriteLine(input1 + math + input2 + "=" number1 + number2);
Thanks ~James