Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial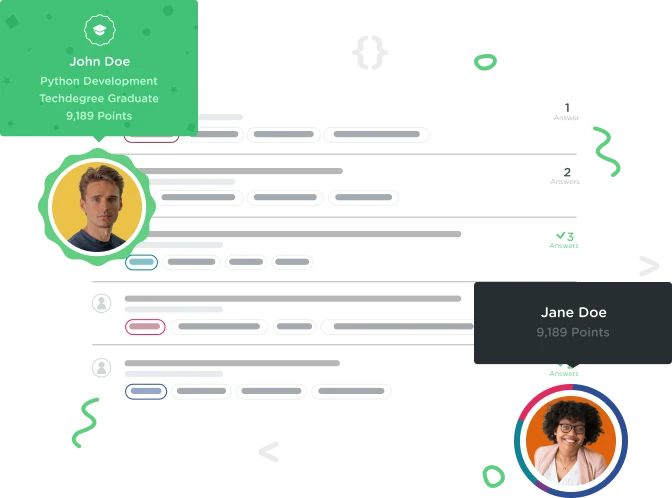
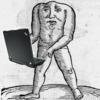
nicholasgryczewski
2,919 PointsUse an escape character to add a newline to the end of the string.
Use the $fullname variable to display the following string to the screen. Use an escape character to add a newline to the end of the string.
'Rasmus Lerdorf was the original creator of PHP'
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullname = "$firstName $lastName";
echo "$fullname\n was the original creator of PHP"
?>
5 Answers

andren
28,558 PointsThe problem is that you have placed the newline character after the $fullname
variable, not at the end of the string. The string ends at the closing quote mark. So it should look like this:
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullname = "$firstName $lastName";
echo "$fullname was the original creator of PHP\n"
?>

Roger Dailey
14,887 PointsIt looks like you forgot to use the concatenation( I hope I spelled that right..) symbol " . " Also you need to put the newline break at the end. This is how I completed the challenge...
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullname = "$firstName $lastName";
echo $fullname . ' was the original creator of PHP\n';
?>

stevenpurvis
3,485 PointsYou don't need the concatenation operator since the example was using double quotes. The reason that andren's example didn't work for you is that it's missing the semicolon on the end of the string. Also the newline character \n would be appear as '\n' literally within single quotes rather than an actual new line.
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullname = "$firstName $lastName";
echo "$fullname was the original creator of PHP\n";
// works the same as
echo $fullname . 'was the original creator of PHP' . "\n" ;
//this will not work
echo $fullname . 'was the original creator of PHP\n';
?>

andren
28,558 PointsSteven Purvis: To quote the official PHP documentation:
The closing tag of a block of PHP code automatically implies a semicolon; you do not need to have a semicolon terminating the last line of a PHP block.
Since the semicolon is used to separate PHP statements from each other it is considered to be optional for your last line of code, since there is no statement that need to be separated from it.
It is indeed good practice to include it regardless, but the omission of that semicolon is not what caused Nicholas to not be able to finish the task with my code. As stated I verified the code before posting it. And after Nicholas complained I also ran it locally on my machine just to double check, and the code ran without any problems.
I also ran it though 3v4l which is a site that allows you to test PHP code compatibility and performance by running it on 200 different versions of PHP and verifying that the code runs successfully on all of them. You can see the result of running that here.
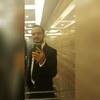
Aman Mishra
8,368 PointsCapitalise the 'N' in full name variable like $fullName. It will let the echo command use the full name variable. Hope this will fix the issue.
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullName = $firstName . " " . $lastName;
echo "$fullName was the original creator of PHP\n"
?>

rahul chopra
Courses Plus Student 466 PointsTry this out 100% working
<?php
$firstName = "Rasmus";
$lastName = "Lerdorf";
$fullName = $firstName .' '. $lastName ;
echo $fullName. ' was the original creator of PHP'. "\n";
?>
nicholasgryczewski
2,919 Pointsnicholasgryczewski
2,919 Pointsdidn't work.
andren
28,558 Pointsandren
28,558 PointsDid you copy the code exactly? I verified that the solution was accepted before I posted it. The code checker for these challenges tend to be very picky so if there is anything off in your string at all then it will often mark your code as wrong.
The code challenges can be a bit buggy at times though, in the worst case scenario you can just restart the challenge and copy and paste the code. That should definitively work.