Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial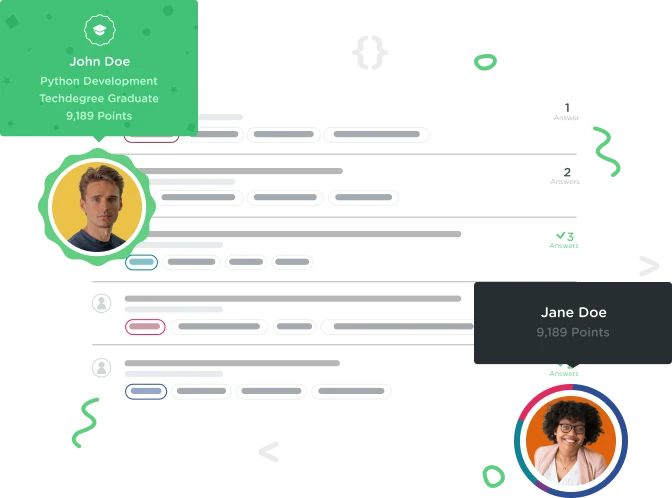
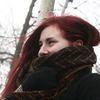
Ella Ruokokoski
20,881 PointsA problem understanding the reducer syntax
Hi! I got a job as a trainee in a tech company and they use React with Redux. I have the basic knowledge of react and have been studying using it with Redux, already build a simple calculator app with them. My problem is understanding how to return the new state when the initialState contains multiple arrays with objects in them.
for example, my initialState looks like this:
const initialState = {
colors: [
{
color: 'black',
active: 'selected',
},
{
color: 'red',
active: '',
},
{
color: 'blue',
active: '',
},
{
color: 'yellow',
active: '',
}
],
sliders: [
{
name: 'red',
value: 0,
},
{
name: 'green',
value: 0,
},
{
name: 'blue',
value: 0,
}
]
}
// here's my snippet from my reducer file
switch(action.type) {
case ColorActionTypes.TOGGLE_COLOR:
return state.colors.map((color) => {
if (color.active === 'selected') {
return
// here the color objects should have their active property's value changed to ''
}
if(color.color === action.color && color.active === '') {
return // here it should change the active property's value to 'selected'
}
return color
})
I have an action called TOGGLE_COLOR that is supposed to toggle the 'selected' class between the colors when clicked. I can make with work if my initial state is only one array with the color objects, but I have trouble understanding what should the return statement look like when my initialState is an object with multiple arrays that contain objects. I just can't seem to get my head around it because I'm only starting with Redux. Also, I am wondering should the reducer only be one file with just one initial state or can there be multiple reducer files? Can anybody give me a syntax example to clarify it for me, please? Would greatly appreciate it.
1 Answer
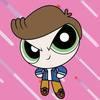
Cezary Burzykowski
18,291 PointsHello Ella!
I have only few minutes now so I will only briefly adress your questions but if you will have any more just let me know.
So to start with you can (and with bigger apps you should) have more then one reducer. We should typicaly have one reducer for every group of actions. For example here we have reducer for all the actions concerning players I we would like to put Stopwatch into Redux aswell it should go into another file.
There is a simple reason for this, each reducer will return its own part of state. So if you would have two reducers:
playerReducer and stopwatchReducer you application state would look someway like this:
state = {
playerReducer: { here goes all the data connected to player }
stopwatchReducer: { here goes all the data connected to stopwatch }
}
When it comes to return statement in Reducers, the easiest way to learn (and rember) is that it should always return your current state aswell as changes. So you should get something like this:
return {...initialState, {name: 'blue', active: true }};
Although it gets a little messy in a way this is organised. I would personally create a separate reducer called 'selectedColorReducer' where I would hold the id / value of selected colors. Then on the component level I would compare selectedColorId with the colorId and change the rendering according to that.
If you will have any further questions just let me know,
Cezary