Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial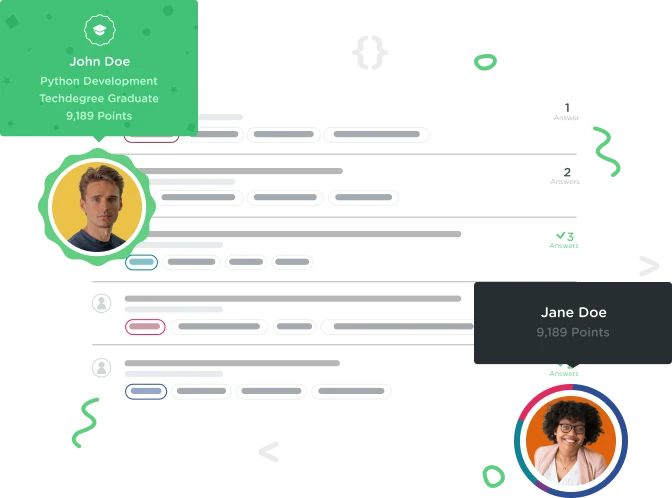

James Croxford
12,723 PointsAccessing object property values using dot notation and brackets- clarification on when brackets must be used?
For this class a for-loop was created inside the callback function to process an XML HTTP request. This callback functions runs through the following data string, converted it to a JavaScript array of objects, and then creates a bit of HTML stating whether each employee is in or out of the office.
[
{
"name": "Aimee",
"inoffice": false
},
{
"name": "Amit",
"inoffice": false
},
{
"name": "Andrew",
"inoffice": true
},
{
"name": "Ben",
"inoffice": true
},
//etc etc
I set up my for looping using a template literal like so:
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
let employees = JSON.parse(xhr.responseText);
let statusHTML = `<ul class="bulleted">`;
for (let i = 0; i < employees.length; i++) {
if (employees[i]['inoffice'] === false) {
statusHTML += `<li class="out">${employees[i]['name']}</li>`;
} else {
statusHTML += `<li class="in">${employees[i]['name']}</li>`;
}
}
statusHTML += `</ul>`;
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
Unlike Dave, I used bracket notation to access the property values of each property within each object. I did this because of the earlier JS Objects course, which explained that running through the values of an object, you should use bracket notation over dot notation whilst in a 'for-in' loop, like this:
for (let property in person) {
console.log(`${property}: ${[property]}`);
}
//returns:
// name: Edward
// nickname: Duke
// etc etc
//i.e. values are dynamically filled in
//NOTE: we CAN'T do this:
for (let property in person) {
console.log(`${property}: ${person.prop}`);
}
//As the console then outputs undefined for each value:
//Returns:
// name: undefined
// nickname: undefined
// etc etc
I think I got a bit confused by this. Am I right in thinking that using brackets only (not dot notation) applies only to this case when we are using a for-in loop on an object, and not in other cases such as the one presented in the class?