Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial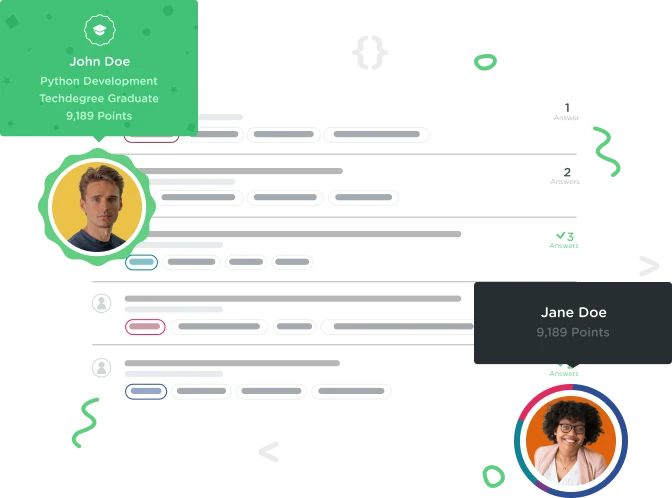

Brenda Krafft
18,036 PointsAll of my attempts to fetch data from the results of my database query give me errors.
I am using mySQL. Not matter what syntax I use, I can not fetch the results of my query.
Connecting to the database works just fine.
Then I create a PDO:
//***************************************************************
// Create a new PDO instance (connection between PHP and server)
//***************************************************************
try {
$db = new PDO($dsn, $user, $password);
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
var_dump($db);
echo "SUCCESS!!";
//die(); //to test
} catch(Exception $e) {
echo $e->getMessage();
die();
}
This also works just fine.
But when I try to query the database, no matter what I do I get all kinds of errors. (See specifics in the comments.) I know that my results returned fine, because when I var_dump them I see the array and it has data.
//***********************************************
// Try to retrieve list of states
//**********************************************
try {
$result = $db->query('SELECT * FROM state');
echo '<pre> Test 1';
//$test1 = $result->fetch_array(MYSQLI_NUM); //gives error: undefined method 'fetch_array()''
echo 'Test 2';
//$test2 = $result->fetch(PDO::FETCH_ALL); //gives error: Undefined class constant 'FETCH_ALL'
echo 'Test 3';
// $test3 = $result->fetch_all(); //gives error: undefined method 'fetch_all()'
echo 'Test 4';
//$test4 = $mysqli->fetch_all(); //gives error: undefined method 'fetch_all()'
echo 'Test 5';
$test5 = $result->fetch_assoc(); //gives error: undefined method 'fetch_assoc()'
echo '</pre>';
} catch(Exception $e) {
echo $e->getMessage();
die();
}
Am I way off here? Some things I've read on other forums indicate problems with the version of php, but I just downloaded the latest this week. Am running version 5.6.12.

Brenda Krafft
18,036 PointsGr.... So I tried Chris's suggestion using fetchAll(), and I still got the "undefined method" error. Used exact same syntax with constant passed in and everything.
Chris are you also using mySQL for your server?
1 Answer
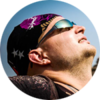
Riku S.
11,322 Points$db is your PDO connection variable, so like this you can get fetchAll done properly:
$sql = $db->prepare("SELECT * FROM state");
$sql->execute();
$result = $sql->fetchAll(PDO::FETCH_ASSOC);

Brenda Krafft
18,036 PointsThat was it! Thank you SO much!
Chris Coleman
5,579 PointsChris Coleman
5,579 PointsThe command you are looking for is fetchAll().
I have code like: $result = $sql->fetchAll(PDO::FETCH_ASSOC);