Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial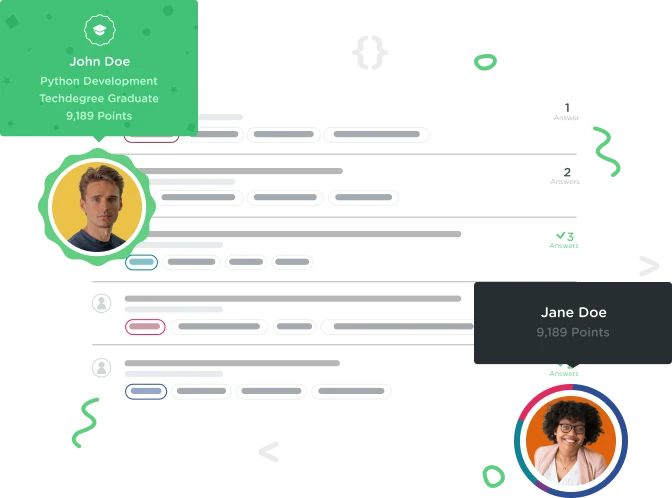

Dylan Anderson
506 PointsAndroid Development Stage 2 Incrementing and decrementing Challenge syntax errors
this returns a whole bunch of syntax errors
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean charge() {
boolean isFullyCharged = false;
if (!isEmpty()) {
mBarsCount--;
charge = true;
}
return charge;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
3 Answers
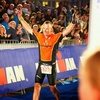
Steve Hunter
57,712 PointsRight - let's have a crack at this. I have the challenge opn and can see the first task is to use a while
loop within charge
so tht the battery will only charge until the battery reports being fully charged. It is saying to use a !
somewhere, which is a good hint.
At the moment, charge
does very little - it just sets the member variable mBarsCount
to be equal to MAX_ENERGY_BARS
. That's a bit blunt, so we're going to refine that. Reading the question lets us know that we want to increment mBarsCount
within charge
using a while
loop. We want to do that until the battery is fully charged and we have a method called isFullyCharged
to let us know when that is.
Right ... how about:
public void charge() {
while(!isFullyCharged()){
mBarsCount++;
}
}
So, the while
loop is looking for a true/false
answer to decide whether it loops or not. So it is looking at isFullyCharged
to see if that is or isn't fully charged. We use the !
to negate the test as if the kart IS fully charged, we don't want it to loop but if it ISN'T, we do. So, while isFullyCharged
returns false
the loop runs. Within the loop, we increment, add one to, mBarsCount
and then we repeat the test. So, again, is the kart isn't fully charged, we go round the loop again and incremenet mBarsCount
until we finally get to the point where !isFullyCharged
is false, i.e. it is fully charged!
And we're done!
Let me know if that's OK - did the reasoning make sense?
Thanks,
Steve.
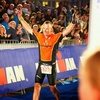
Steve Hunter
57,712 PointsHi Dylan,
I'm not sure what the aim is here. It would help if you could let us know what you're trying to solve and where your errors are.
public boolean charge() {
boolean isFullyCharged = false;
if (!isEmpty()) {
mBarsCount--;
charge = true;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
For example, you have two methods called the same. What does the charge
method need to do?
I've had a few queries on this one, so there's some forum help out there:
https://teamtreehouse.com/forum/completely-lost-3 https://teamtreehouse.com/forum/illegal-start-of-type-for-while-loop
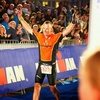
Steve Hunter
57,712 PointsHi Dylan,
I'm happy to take you through this one bit by bit but I don't have the chance to do that tonight.
Is it OK if I post an answer tomorrow going over the challenge and trying to explain what I'm on about!?
As I say, I have other commitments tonight so can't do it now.
Steve.
Dylan Anderson
506 PointsDylan Anderson
506 Points./GoKart.java:13: error: class, interface, or enum expected public boolean dispense() { ^ ./GoKart.java:15: error: class, interface, or enum expected while (!isEmpty()) { ^ ./GoKart.java:17: error: class, interface, or enum expected wasDispensed = true; ^ ./GoKart.java:18: error: class, interface, or enum expected } ^ ./GoKart.java:20: error: class, interface, or enum expected } ^ ./GoKart.java:23: error: class, interface, or enum expected public String getColor() { ^ ./GoKart.java:25: error: class, interface, or enum expected } ^ ./GoKart.java:27: error: class, interface, or enum expected public void charge() { ^ ./GoKart.java:29: error: class, interface, or enum expected } ^ ./GoKart.java:31: error: class, interface, or enum expected public boolean isBatteryEmpty() { ^ ./GoKart.java:33: error: class, interface, or enum expected } ^ ./GoKart.java:35: error: class, interface, or enum expected public boolean isFullyCharged() { ^ ./GoKart.java:37: error: class, interface, or enum expected } ^ JavaTester.java:58: error: cannot find symbol if (!kart.isBatteryEmpty() || kart.isFullyCharged()) { ^ symbol: method isBatteryEmpty() location: variable kart of type GoKart JavaTester.java:58: error: cannot find symbol if (!kart.isBatteryEmpty() || kart.isFullyCharged()) { ^ symbol: method isFullyCharged() location: variable kart of type GoKart JavaTester.java:62: error: cannot find symbol kart.charge(); ^ symbol: method charge() location: variable kart of type GoKart JavaTester.java:63: error: cannot find symbol if (!kart.isFullyCharged()) { ^ symbol: method isFullyCharged() location: variable kart of type GoKart 17 errors
these are the errors