Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial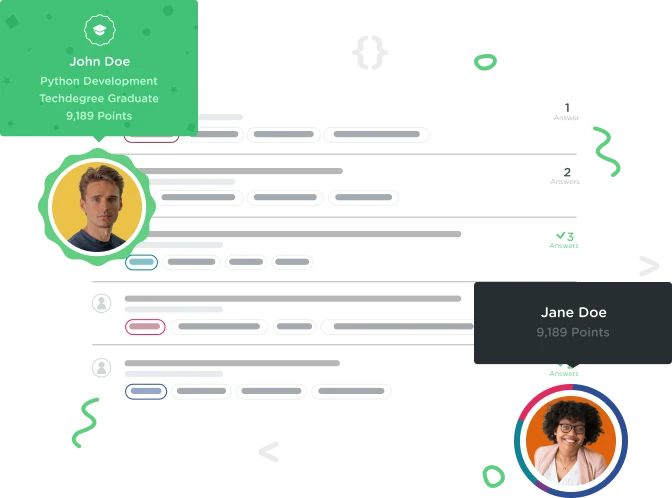
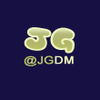
Jonathan Grieve
Treehouse Moderator 91,253 PointsAndroid Extra Credit - getting user input
Here's my code from my extra credit attempt for the Interactive Story course. As you can see, it generates a scary runtime error when I attempt to tab the button associated with the EditText.
Or if you want to clone it on Github, here it is! https://github.com/jg-digital-media/android-user-input
My situation at the moment is I'm very stuck with my Android learning having completed the first 3 apps in the track. The Facts app, the story and the weather app.
In the main, it's got nothing to do with understanding what's been taught. The teaching is on point and superb. I'm afraid it's just my short term memory. My brain isn't built for remembering blocks of code. So, instead of committing things to memory, I of course, look up documentation where I can and keep snippets of code I deem helpful.
But is that enough?
The Extra credit task is simply to get text from different Android classes (EditText, RadioButton) etc and log it to the console or as a toast.
I wanted to tackle all of this on my own, but basically, I don't really know what I'm doing.
package uk.co.jonniegrieve.androiduserinput;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.Spinner;
import android.widget.Switch;
import android.widget.Toast;
import android.widget.ToggleButton;
import static uk.co.jonniegrieve.androiduserinput.R.id.editTextButton;
public class MainActivity extends AppCompatActivity {
//declare variables
private EditText mEditText;
private CheckBox mCheckBox;
private RadioButton mRadioButton;
private Switch mSwitch;
private ToggleButton mToggleButton;
private Spinner mSpinner;
private Button editTextButton;
private Button checkboxButton;
private Button radioButton;
private Button switchButton;
private Button toggleButton;
private Button spinnerButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mEditText = (EditText) findViewById(R.id.editText);
mCheckBox = (CheckBox) findViewById(R.id.checkbox);
mRadioButton = (RadioButton) findViewById(R.id.radioButton);
mSwitch = (Switch) findViewById(R.id.switchView);
mToggleButton = (ToggleButton) findViewById(R.id.toggleButton);
mSpinner = (Spinner) findViewById(R.id.spinner);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
//code goes here
String name = mEditText.getText().toString();
Toast.makeText(MainActivity.this, name, Toast.LENGTH_LONG).show();
}
};
editTextButton.setOnClickListener(listener);
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
android:id="@+id/activity_main"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="uk.co.jonniegrieve.androiduserinput.MainActivity"
tools:background="#68ff6d">
<TextView
android:text="Getting User Input TextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/inputTextView"
android:textAlignment="center"
android:textStyle="normal|bold"
android:textColor="#459b14"
android:layout_below="@+id/mainTitleTextView"
android:layout_centerHorizontal="true"/>
<TextView
android:text="Textbox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="11dp"
android:layout_marginStart="11dp"
android:layout_marginTop="29dp"
android:id="@+id/textView2"
android:layout_below="@+id/inputTextView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"/>
<TextView
android:text="Radio Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/Checkbox"
android:layout_alignLeft="@+id/Checkbox"
android:layout_alignStart="@+id/Checkbox"
android:layout_marginTop="27dp"
android:id="@+id/textView4"/>
<TextView
android:text="Toggle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/Switch"
android:layout_alignLeft="@+id/Switch"
android:layout_alignStart="@+id/Switch"
android:layout_marginTop="32dp"
android:id="@+id/Toggle"/>
<TextView
android:text="checkbox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:id="@+id/Checkbox"
android:layout_below="@+id/textView2"
android:layout_alignLeft="@+id/textView2"
android:layout_alignStart="@+id/textView2"/>
<CheckBox
android:text="CheckBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/checkBox2"
android:layout_alignBaseline="@+id/Checkbox"
android:layout_alignBottom="@+id/Checkbox"
android:layout_toRightOf="@+id/textView4"
android:layout_toEndOf="@+id/textView4"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/checkBox2"
android:layout_alignLeft="@+id/editTextButton"
android:layout_alignStart="@+id/editTextButton"
android:id="@+id/checkboxButton"/>
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:text="Name"
android:ems="10"
android:id="@+id/editText"
android:layout_alignBaseline="@+id/textView2"
android:layout_alignBottom="@+id/textView2"
android:layout_toLeftOf="@+id/editTextButton"
android:layout_alignLeft="@+id/inputTextView"
android:layout_alignStart="@+id/inputTextView"/>
<RadioButton
android:text="RadioButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/checkboxButton"
android:layout_toRightOf="@+id/textView4"
android:layout_toEndOf="@+id/textView4"
android:layout_marginLeft="14dp"
android:layout_marginStart="14dp"
android:id="@+id/radioButton"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/radioButton"
android:layout_alignLeft="@+id/radioButton"
android:layout_alignStart="@+id/radioButton"
android:id="@+id/switchButton"/>
<ToggleButton
android:text="ToggleButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/toggleButton"
android:layout_alignBaseline="@+id/Toggle"
android:layout_alignBottom="@+id/Toggle"
android:layout_alignRight="@+id/checkBox2"
android:layout_alignEnd="@+id/checkBox2"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/toggleButton"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:layout_below="@+id/switchButton"/>
<TextView
android:text="Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/Switch"
android:layout_below="@+id/radioButton"
android:layout_alignRight="@+id/Checkbox"
android:layout_alignEnd="@+id/Checkbox"
android:layout_alignLeft="@+id/textView4"
android:layout_alignStart="@+id/textView4"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ANDROID PROJECT"
android:id="@+id/mainTitleTextView"
android:textAlignment="center"
android:textStyle="normal|bold"
android:textSize="30sp"
android:textColor="#459b14"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"/>
<TextView
android:text="Spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/toggleButton"
android:layout_alignLeft="@+id/Toggle"
android:layout_alignStart="@+id/Toggle"
android:id="@+id/textView9"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/editTextButton"
android:layout_alignBaseline="@+id/editText"
android:layout_alignBottom="@+id/editText"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"/>
<Switch
android:text="Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/switchView"
android:layout_alignTop="@+id/Switch"
android:layout_alignLeft="@+id/editText"
android:layout_alignStart="@+id/editText"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/radioButton"
android:layout_below="@+id/checkboxButton"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"/>
<Button
android:text="Show Value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/spinnerButton"
android:layout_below="@+id/toggleButton"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"/>
<Spinner
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/spinner"
android:layout_alignBottom="@+id/spinnerButton"
android:layout_alignRight="@+id/textView9"
android:layout_alignEnd="@+id/textView9"/>
</RelativeLayout>
1 Answer

Seth Kroger
56,413 PointsI think you have a good start on it. I cloned your repo and ran it. It looks like the app is crashing because you're forgetting to initialize the Show Value buttons as well as the inputs. That leaves them null, hence a NullPointerException when you try to set a listener. The second thing that's really easy to miss in all the scrolling is there are two stack traces that get spit out. Before the actual crash you get:
>>>
03-16 15:05:54.829 1921-1921/com.android.launcher3 E/CellLayout: Ignoring an error while restoring a view instance state
>>>
java.lang.IllegalArgumentException: Wrong state class, expecting View State but received class android.appwidget.AppWidgetHostView$ParcelableSparseArray instead. This usually happens when two views of different type have the same id in the same hierarchy. This view's id is id/0x4. Make sure other views do not use the same id.
Looking at your layout you're using 'radioButton' as as the id for both the RadioButton and it's Show Value button, and likewise with 'toggleButton'.
Yeah, Android stack traces can be pretty scary, but it gets better with practice. The two big things to look for are the actual exception message and the first point your code instead of Android code shows up. Most of the stack trace beyond those two pieces is usually irrelevant.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsThanks for your responses. :)
When I say "I don't know what I'm doing" clearly I know some things because and I have knowledge in my brain. It's just what happens when I'm let "loose in the wild" so to speak. It's a huge corner I've yet to turn
So the first things to try are Initialise "Show Value" buttons
Look at the id's for radio button and toggleButtons and make them unique
Hopefully after that, I'm pretty confident I can incrementally (for want of a better word) add the toasts for each input one by one.
I'll let you know how I'm getting on but I'm downloading AS 4.3 and have major updates that are taking an age to come through! :)
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsWell I've just pushed up my latest changes, getting text from the EditText. Looking forward to learning how to get input from the rest of the controls.
Thanks for the nudge! :)