Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial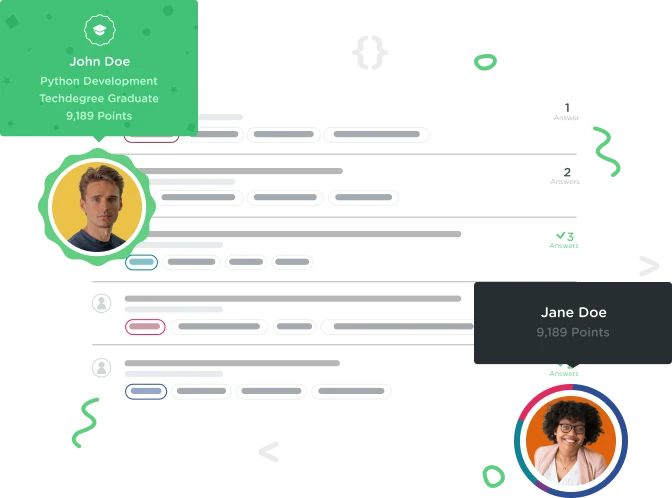
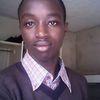
danielkimeli
1,196 PointsAnother Go interface solution I can get past
In the clock and calendar packages, we've defined Clock and Calendar types, both of which have a Display method that you can call to print them. In the schedule package, define a Displayable interface that is satisfied by the Display methods on both the Clock and Calendar types. (Don't make any changes to the clock or calendar packages.) Then, still in the schedule package, define a Print function that takes a Displayable value and calls Display on it.
package schedule
type Displayable interface {
Display() int
}
func Print (display Displayable) int {
d := display.Display()
return d
}
package clock
import "fmt"
type Clock struct {
Hours int
Minutes int
}
func (c Clock) Display() {
fmt.Printf("%02d:%02d", c.Hours, c.Minutes)
}
package calendar
import "fmt"
type Calendar struct {
Year int
Month int
Day int
}
func (c Calendar) Display() {
fmt.Printf("%04d-%02d-%02d", c.Year, c.Month, c.Day)
}
3 Answers
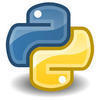
Gerald Wells
12,763 PointsA concrete type specifies exact representation of its values and exposes intrinsic opertations.
An interface on the other hand is an abstract type. It doesn't expose the representation or internal structure of its values or the set of basic operations they support; it reverals only some of their methods.
In saying this we can infer that the interface itself only needs to know what methods will be used not the context behind them. You can abstract that one more layer and write and interface for an interface(but I won't get into that).
package schedule
// DECLARE A Displayable INTERFACE HERE
type Displayable interface {
Display()
}
The function is calling d
with a type of Displayable
interface.
// DECLARE A Print FUNCTION HERE
func Print( d Displayable) {
d.Display()
}
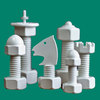
Steven Parker
230,069 PointsIn your interface, you define "Display()" as returning an "int". But if you look at the function implementations in the other files, you will see that neither one has any return value.
Then, your "Print" function is also defined to return an "int", and in the code it gets it from calling "Display()". But since that call does not actually return a value you won't be able to assign it to anything. And you probably don't need to make "Print" return anything, either.
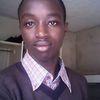
danielkimeli
1,196 PointsThank you, your explanation was very clear, and with the help of the code below I managed it.
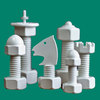
Steven Parker
230,069 PointsI intentionally did not provide code because I think most people have a better learning experience if they complete it themselves with a few hints.
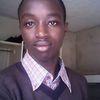
danielkimeli
1,196 PointsI know, I applied it here https://teamtreehouse.com/community/i-cant-find-a-solution-to-struct-challenge
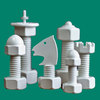
Steven Parker
230,069 PointsSo on this question?
danielkimeli
1,196 Pointsdanielkimeli
1,196 PointsThanks this actually worked, I have know where i went wrong on from your explanation and >Steven Parker's above thanks again