Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial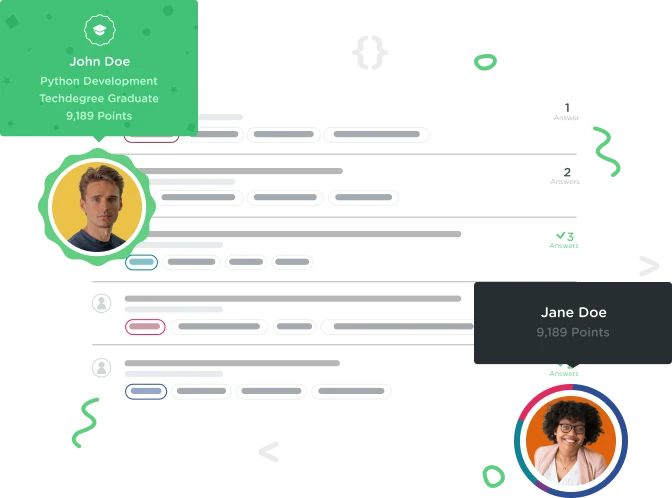

Parsa Khodabakhshi
1,024 PointsApp Crashes after clicking Show another fact button
I am using android studio 3.6.2 I had an earlier problem as well where I couldn't find the R file that contained the id's but I assumed it exists and moved on. I have the same code as Ben, and there are no red lines or errors but when I run the app on the emulator it works until I click the show another fact button then the application just closes, but no errors come up in the even log. I have included my MainActivity.java file below and the activity_main.xml below as well.
xml code:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#51B46D" android:padding="50dp" tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Did You Know?"
android:textColor="#80FFFFFF"
android:textSize="24sp" />
<TextView
android:id="@+id/funFactText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:text="Ants strech when they wake up in the morning."
android:textColor="#FFFFFF"
android:textSize="24sp" />
<Button
android:id="@+id/showFactButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentBottom="true"
android:layout_marginBottom="0dp"
android:background="@android:color/background_light"
android:text="Show Another Fact"
android:textSize="18sp"
android:layout_alignParentLeft="true" />
</RelativeLayout>
Main java code
package appExample.com;
import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class MainActivity extends AppCompatActivity { // Declare View variables private TextView factTextView; private Button showFactButton; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
// Assign the Views from layout file to the corresponding variables
factTextView = (TextView) findViewById(R.id.factTextView);
showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
// The button was clicked so update the fact TextView with a new fact
String fact = "Ostriches can run faster than horses";
factTextView.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}