Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial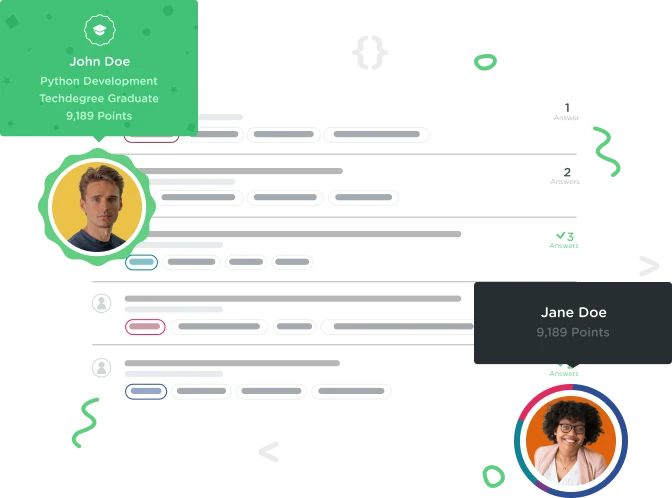

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsArrow Function - ES6, I am a little stuck any hint or suggestion is very much appreciated thank you.
Task:
convert the function in the arrowfunction.js file into ES6 functions
JS CODE:
function addFive(num) { return num + 5; } // TODO: Convert addFive() to arrow function let addFive = (num) => { num + 5; };
function divideBy(x, y) { return x / y; } // TODO: Convert divideBy() to arrow function let divideBy = (x, y) => { return x / y; };
function logToConsole() { let msg = "MIT Coding Certificate"; console.log(msg); } // TODO: Convert logToConsole() to arrow function let logToConsole = () => { let msg = "MIT Bootcamp"; console.log(msg); };
1 Answer

Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsWell, you were very close.
- You cannot have to functions with the same name so I renamed the arrow function.
- If you use the { } around the function body it needs a return statement. See two examples below.
So I reworked you code as follows:
function addFive(num){ return num + 5; }
const add_Five = (num) => num + 5; ;
const add__Five = (num) => { return num + 5; };
console.log(addFive(10));
console.log(add_Five(10));
console.log(add__Five(10));
// TODO: Convert divideBy() to arrow function
function divideBy(x, y) { return x / y; }
let divide_By = (x, y) => { return x / y; };
console.log(divideBy(20,5));
console.log(divide_By(20,5));
// TODO: Convert logToConsole() to arrow function
function logToConsole() { let msg = "MIT Coding Certificate"; console.log(msg); }
let logTo_Console = () => { let msg = "MIT Bootcamp"; console.log(msg); };
logToConsole();
logTo_Console();
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointsthank you sir Michael Kobela