Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial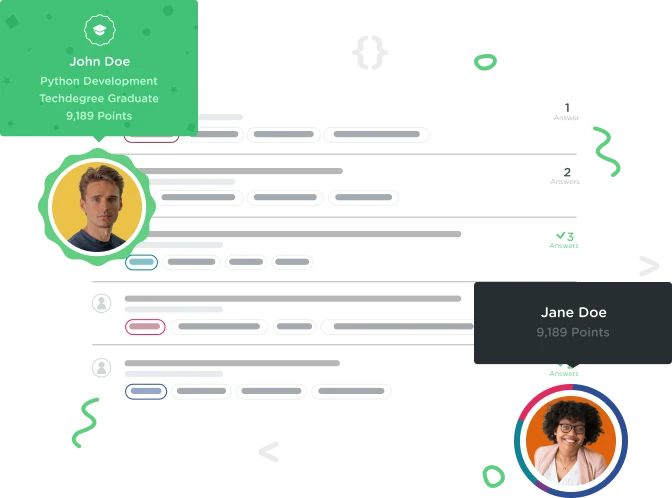
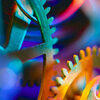
ja5on
10,338 Pointsarrow function or variable?
var arrowTotal = () => multiply() + add();
document.querySelector("h3").innerHTML = <h3>This is the sum total of both ARROW functions <strong>${arrowTotal()}.</strong></h3>
;
With the arrow function, am I calling the arrow function itself or am I calling the variable? Seems to me like both, but In regards of tech speak would I say I'm calling the arrowTotal function which just happens to be stored into a variable??
3 Answers
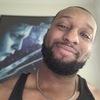
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi jasonj7,
What you've created is a function expression. arrowTotal is the name of your function. You would say you're calling/invoking the arrowTotal function.
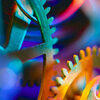
ja5on
10,338 PointsIt feels like I have two people saying two different things, because with var arrowTotal = () => multiply() + add();
the tutoring videos call this a arrow function the () => make it easy to see, I've not been trained in treehouse to see it as a function expression. Please note my experience points are 5228 so you may have come across more advanced information that I'm have not been subject to yet.
So far in my experience the treehouse videos I've seen teach three main types of functions, function declaration, expressions, and arrow.
If I start saying arrow functions are expressions then one bleeds into the other and I cant differentiate between them, this obviously causes more problems than cures.
If your way is right I just haven't come across that at all not even in the function teaching module but maybe in the near future who knows.
As always thank for your continued replies though, they are of value and make me think.
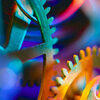
ja5on
10,338 PointsSorry I didn't get what you were saying in your last response and upon further research I found this https://teamtreehouse.com/library/introducing-arrow-function-syntax this video stands outside the JavaScript track I've been on so far. It's helped a lot as I lack some understanding and I guess that there is much more to JavaScript than what I have seen which if I may say your code has been pushing me towards that idea. I have been looking at w3schools and mdn and found more on functions, now I'm a little bit more educated I can see what you mean In your response.
ja5on
10,338 Pointsja5on
10,338 PointsHere is a arrow function or fat arrow function. var arrowTotal = () => multiply() + add();
This is an arrow function. https://www.w3schools.com/js/js_arrow_function.asp hello = () => "Hello World!";
A function expression. https://www.w3schools.com/js/js_function_definition.asp var x = function (a, b) {return a * b};
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsYou're correct that what you wrote is also an arrow function. What makes the second example a function expression is the fact that it's set to a variable, not that it includes the keyword function. So while
var x = function (a, b) {return a * b}
is a function expression,const x = (a, b) => a * b
is also a function expression.I originally mentioned that it was a function expression because you wanted to know if you were calling a function or a variable, and so I wanted to explain that what you did was/is a valid way of crafting a function (for me it's the preferred method, unless I need my function to be hoisted (or I need the
this
keyword to be bound to the function).In fact, if you were to write the following:
What you would see in your console after running that code is the following:
[Function: addTwo]
Your console would classify it as a function.
There are two main ways to write/define a function.
They are via a function declaration and via a function expression. A function declaration uses this syntax:
function addTwo(num) { return num + 2}
A function expression uses the aforementioned syntax, which uses the
var/let/const = functionName(){} OR var/let/const = () => {}
to create a function.Does that make sense?