Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial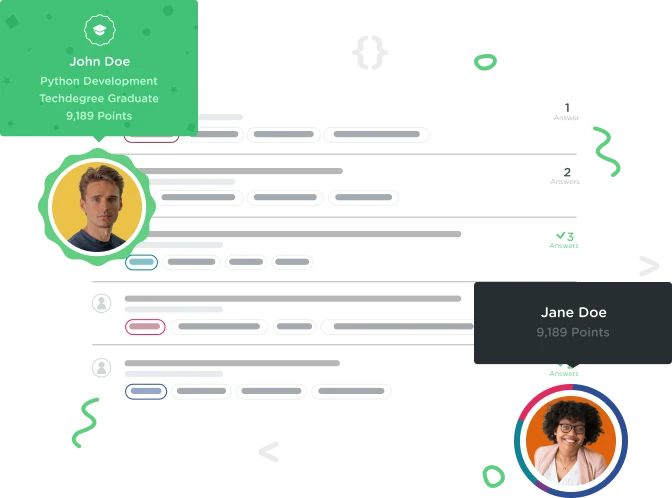

Steven Hughes
17,974 PointsASP.NET MVC Basics challenge 2 of 2 GetVideoGame. Why it is not accepting my foreach loop?
The code keeps getting errors citing the "in" and unexpected ")". I think I may have been looking at it too long because I am not seeing anything mistyped in the code. What did I do wrong?
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public VideoGame[] GetVideoGames()
{ return _videoGames; }
// TODO Add GetVideoGame method
public VideoGame[] GetVideoGame(int id)
{
VideoGame pullVideoGame = null;
foreach(var VideoGame in _videoGames)
{
if(VideoGame.Id == Id)
{
pullVideoGame = VideoGame;
break;
}
}
return VideoGame;
}
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
2 Answers

Ben Reynolds
35,170 Points- This method returns a single video game, not an array, so the brackets can be removed from the return type
- n the foreach statement I'd avoid naming the loop variable the exact same thing as the return type (camel-case "VideoGame" or choose another name, in mine I just used "game")
- In the if statement we're comparing the game's Id (capitalized) to the method parameter id (lowercase). Using "id" on the right side of the comparison operator should fix that one.
- Your technique of setting up a temporary VideoGame object (pullVideoGame) and using the break statement is valid code and would work, but i would definitely recommend simplifying that by removing that as well as the break statement and just return the game in the if block and returning null if it's never found. This would save a small amount of memory in this example, but if this was used in a real application that might have thousands of games the performance gains can really add up.
Here's the code I used:
public VideoGame GetVideoGame(int id)
{
foreach(VideoGame game in _videoGames)
{
if(game.Id == id)
{
return game;
}
}
return null;
}
I generally don't like to just post the answer but this can be a tricky one for beginners and I don't want you to be stuck for too long. I know what it's like to get to the point you just want to finish a challenge to get it overwith and move on! I highly recommend studying this code for a bit instead on just pasting it in and calling it good but I will leave that up to you :)
You're getting there, keep at it!

Steven Hughes
17,974 PointsThank you so much. The naming convention confused me as I thought the foreach loop needed to search for the object by name, but I do remember from front end using a foreach loop where I created a internal variable called splat because it was used no where else. Thank you so much for the detailed explanation. I will add it to my notes. Alsothe Id == id made a lot of sense.