Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial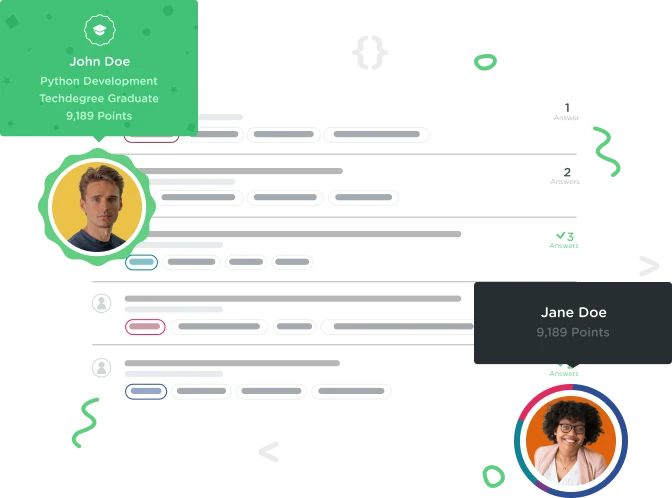
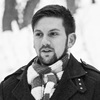
Matthew Gromer
8,334 PointsAssertionError keeps cropping up, repeatedly.
I've tried a number of things, nesting two for statements in what I think should work, but no matter what I do, I keep getting the following error: 'AssertionError: 'join' not found in 'musical_groups =' I'm pretty confident we've not covered joins. What am I missing?
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
# Your code here
for group in len(musical_groups):
for band in len(group):
list += "{}, ".format(musical_groups[group][band])
3 Answers

adrian miranda
13,561 PointsThat doesn't sound like a python error, that sounds more like the treehouse application isn't happy with your answer. I think they want you to use the str.join() function. For example, if you run:
", ".join(list_of_whatever)
python will join all the members of list_of_whatever together, placing ", " between member. Hand that off to print, and it will print them out as a nicely formatted list. Does that give you a better idea of what you need to do?
If you want more help, consider this line:
for group in musical_groups:
What will group contain at that particular point? A list of group members, right? So if you want to print out the members, with a comma and a space between each line, it's pretty simple
for group in musical_groups:
print(", ".join(group))

adrian miranda
13,561 PointsThink carefully about what this does:
for group in len(musical_groups):
What is "len(musical_groups)"? It will give the length of the musical_groups list. That isn't what you want. What you want is to actually take the contents of the list and put them in the variable group. Thus, you want something like:
for group in musical_groups:
That will actually go through the list.
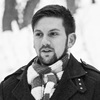
Matthew Gromer
8,334 PointsYeah, I had tried that before, but with similar results. Here's what I'd had before the above example:
# Your code here
for group in musical_groups:
for band in group:
list += "{}, ".format(band)
print(list)
It throws the same error, though. I know I'm missing something here.

adrian miranda
13,561 PointsThink about this line, the first time you execute it:
list += "{}, ".format(band)
What is the "list" variable going to be the first time you run that? Nothing (to be exact, None). You cannot add nothing to a string. Before you start your loop, why don't you set list="" or similar. Then, "list" will be a string, and you can add a string to a string. I'm not sure you'll get the output you want, but it should at least execute.
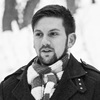
Matthew Gromer
8,334 PointsThat's a great point, I completely forgot about that. I added
list = ""
before the code I had previous, but the same error is being thrown. It's... really confusing. Posting the full error:
File "", line 32, in test_expected_code
AssertionError: 'join' not found in 'musical_groups = [\n ["Ad Rock", "MCA", "Mike D."],\n ["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],\n ["Salt", "Peppa", "Spinderella"],\n ["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],\n ["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],\n ["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],\n ["Run", "DMC", "Jam Master Jay"],\n]\n# Your code here\n\nlist = ""\nfor group in musical_groups:\n for band in group:\n list += "{}, ".format(band)\nprint(list)' : For each group, you should use the join method on a separator to combine the group members
Matthew Gromer
8,334 PointsMatthew Gromer
8,334 PointsAh, that was it, they wanted the join command.