Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial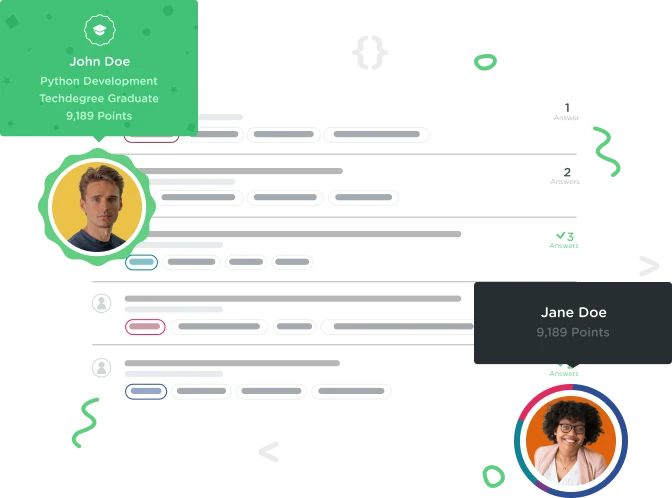
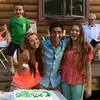
David Axelrod
36,073 PointsAuto Naming reducers?
When we import our reducer into index.js the name is PlayerReducer but in the file it's defined as Player. Does redux handle auto renaming?
Thanks!
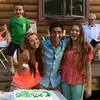
David Axelrod
36,073 PointsHrm interesting. I've done a bit more work with Redux and it does seem like you can import modules as different names if the file only has one export. Can you post your reducer code? Would love to check it out
1 Answer
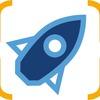
codeboost
6,886 PointsYour Player class is the default export, so you can import it with any name you want. This goes for all the imports not between curly braces. This has nothing to do with Redux, but rather with ES6 exports/imports.
Larisa Popescu
20,242 PointsLarisa Popescu
20,242 PointsI actually have the same question as you, because I get an error saying that "expected the reducer to be a function". Actually I solved it by removing the curly braces from around PlayerReducer reducers/player.js
index.js