Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial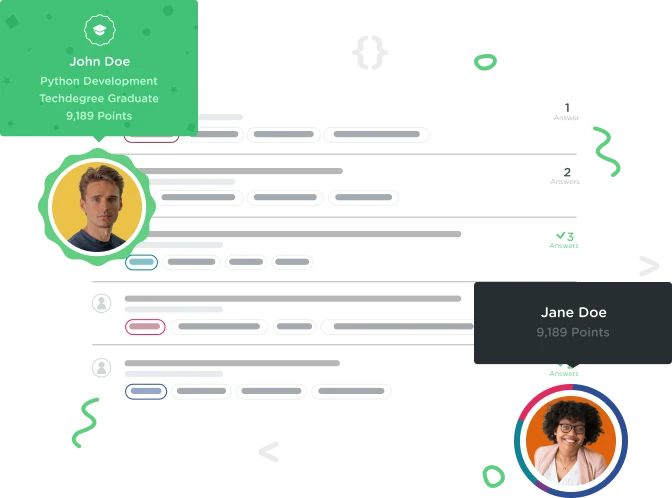
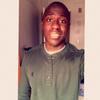
Allan Oloo
8,054 PointsAverage challenge program
I keep getting this scoping error. I am not sure what I am doing wrong. this are the errors I am currently getting. Please help!
Program.cs(26,13): error CS0136: A local variable named `numbertotal' cannot be declared in this scope because it would give a dif
ferent meaning to `numbertotal', which is already used in a `parent or current' scope to denote something else
Program.cs(26,24): error CS1525: Unexpected symbol `+=', expecting `,', `;', or `='
using System;
namespace jenzabar.Average
{
class Progam
{
static void Main()
{
//PROMPT USER FOR A VALUE.
Console.WriteLine("Please enter your name!");
string userName = Console.ReadLine();
Console.WriteLine("Wellcome " + userName + "!");
while(true)
{
double numbertotal = 0;
int numbers = 0;
Console.Write("Please enter a value or enter \"done\" to exit: ");
string userInput = Console.ReadLine();
if (userInput.toLower() == "done")
{
break;
} else {
try
{
var numbertotal += double.Parse(userInput);
numbers++;
} catch(FormatException){
Console.WriteLine("This number is Not valid. Please try again!");
continue;
}
}
Console.WriteLine("The average of the values is: " + numbertotal / numbers);
}
}
}
}
1 Answer

andren
28,558 PointsWhen you use keywords like double
and var
with a variable you are telling C# to create a variable of said type, though var
is technically not a type, but will lead C# to guess the type for you. Since you include those keywords both times you reference the numbertotal
variable you actually tell C# to create the variable twice, which is not allowed.
If you remove the var
keyword from the second mention of numbertotal
like this:
using System;
namespace jenzabar.Average
{
class Progam
{
static void Main()
{
//PROMPT USER FOR A VALUE.
Console.WriteLine("Please enter your name!");
string userName = Console.ReadLine();
Console.WriteLine("Wellcome " + userName + "!");
while(true)
{
double numbertotal = 0;
int numbers = 0;
Console.Write("Please enter a value or enter \"done\" to exit: ");
string userInput = Console.ReadLine();
if (userInput.toLower() == "done")
{
break;
} else {
try
{
numbertotal += double.Parse(userInput); // Removed var keyword
numbers++;
} catch(FormatException){
Console.WriteLine("This number is Not valid. Please try again!");
continue;
}
}
Console.WriteLine("The average of the values is: " + numbertotal / numbers);
}
}
}
}
Then that will fix the current error message, but it's worth noting that there are in fact other issues with your code beyond the one I just pointed out and fixed. Since this is a bonus exercise mainly designed to test your skills I'll let you try to fix those other issues on your own.
You should be able to spot the errors though some debugging on your own, but if you end up not figuring it out you can reply to this post and I'll help you fix the remaining errors.
Allan Oloo
8,054 PointsAllan Oloo
8,054 Pointsits working now, but i am not sure why its not adding new values to previous entered values.
numbertotal += double.Parse(userInput);
this line should add values as user enters new values.
Allan Oloo
8,054 PointsAllan Oloo
8,054 PointsI have changed my code and I am still getting similar issues with the value not adding new entered values. Any suggestion ?
andren
28,558 Pointsandren
28,558 PointsThat's one of the issues I hinted at, that line does add the number to
numbertotal
but at the beginning of the loop you have this line:double numbertotal = 0;
Which sets the
numbertotal
to 0, so even though the number is added to it it is reset as soon as the loop starts, when writing loops you always have to make sure that all of the code placed within it is stuff you actually want to run every time the loop restarts, any code that only needs to run once should always be placed outside the loop.The same issue will also occur with your
numbers
variable. But the issue can be solved by just moving the variable declarations outside of the loop.Edit: I see you figured the issue out already, you posted your revised code just as I posted this.
I can't actually see any issue with the revised code you posted, and running it myself it seems to work fine, what issue is it that you are currently having with it?
Allan Oloo
8,054 PointsAllan Oloo
8,054 Pointsmoved the loop within the try clause. The variables are declared outside of the loop.
andren
28,558 Pointsandren
28,558 PointsYep, I saw that post right after I posted my response, see the edit I attached to the bottom of my previous post.
Allan Oloo
8,054 PointsAllan Oloo
8,054 PointsDoes my current code reflect the variable declaration outside the while? They should not be reseting after the user hits done.
The current Issue is that my code keeps reseting. Not sure why.
andren
28,558 Pointsandren
28,558 PointsThe
numbertotal
is being updated correctly while the program runs, since the loop is the last thing in your program the program exits automatically the user hits done. Variables are used to store data temporarily while a program runs, they do not store data across restarts of the program.What exactly do you want the program to do when the user enters "done"? If you want to print out the average one last time then you can just place a print statement before the
break
in that if statement, but once the program has exited the value won't be stored any longer.Allan Oloo
8,054 PointsAllan Oloo
8,054 PointsYAY! It works. Thank you. Your awesome Lets keep in touch.
Ankit Biswas
280 PointsAnkit Biswas
280 PointsWhy you use number++??