Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial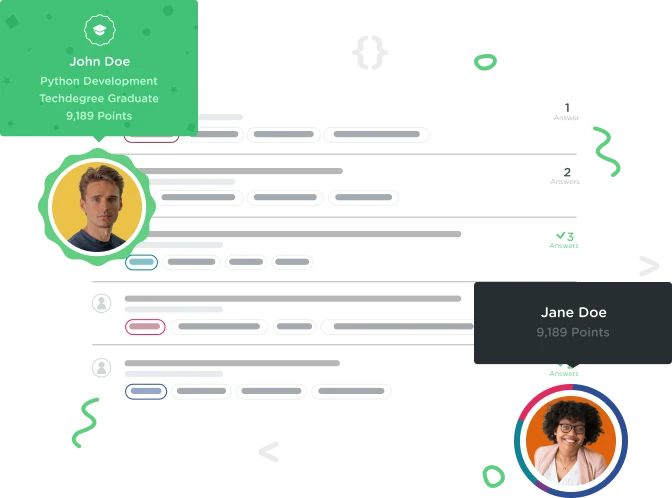

Emily Zachery
10,935 PointsBad Array Declarator
I have tried more than a dozen different configurations of the array declaration and placement, but I keep getting the same error message about bad array declarator. No matter where I put the brackets, what I put in the brackets... nothing seems to make any difference. I've watched the videos a million times. I just don't understand what the hell is going on.
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
int m1= maxFactor+1;
for(int rowIndex = 0; rowIndex < m1; rowIndex++)
{
for(int colIndex = 0; colIndex < m1; colIndex++)
{
int[,] BuildMultiplicationTable1[rowIndex,colIndex] = rowIndex*colIndex;
}
}
return BuildMultiplicationTable1;
}
}
}
2 Answers
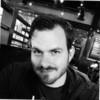
Brent Phillips
Front End Web Development Techdegree Student 12,627 PointsHi Emily,
You are very close to the solution! Allow me to try and help you through it:
The problem, as you pointed out, is in your array declaration: You are trying to initialize the array within the loop, which won't work. Every time the loop hits your array declaration it tries to re-initialize it, over and over again. All of your declarations need to happen outside of the loop. So, declare your array right below your 'm1' declaration. Don't forget to use your 'new' keyword when initializing your array, and remember that we are building a matrix, so your rows and columns will be the same length(Hint: your 'm1' variable will come into play here).
Next, your loop looks great. At the bottom level, we are no longer initializing the array, only modifying it. Drop the 'int[,]' and your logic will work fine. Finally, don't forget to make sure your return statement returns the correct name of your array.
Give it another shot and let me know how it goes.
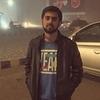
zainsra7
3,237 PointsEmily the thing is that you're creating BuildMultiplcationTable1 Multidimentional Array inside inner for loop so its scope is limited to that and outside the { } braces of inner for loop it can't be accessed.
Secondly , before using such arrays You need to declare and initialize them with "new" keyword.
So in order to make it work, Do following steps:
- Initialize your Array properly outside the loops , after the function.
int[,] BuildMultiplicationTable1 = new int[4,3]; //It will create an array of 4 rows and 3 columns
- Use the above created array just like a normal variable, rest of your code works perfect !
Here's your modified code and it works in the challenge now !
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
int m1= maxFactor+1;
int[,] BuildMultiplicationTable1 = new int[maxFactor+1,maxFactor+1];
for(int rowIndex = 0; rowIndex < m1; rowIndex++)
{
for(int colIndex = 0; colIndex < m1; colIndex++)
{
BuildMultiplicationTable1[rowIndex,colIndex] = rowIndex*colIndex;
}
}
return BuildMultiplicationTable1;
}
}
}
I hope it answers your question , Happy Coding !