Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial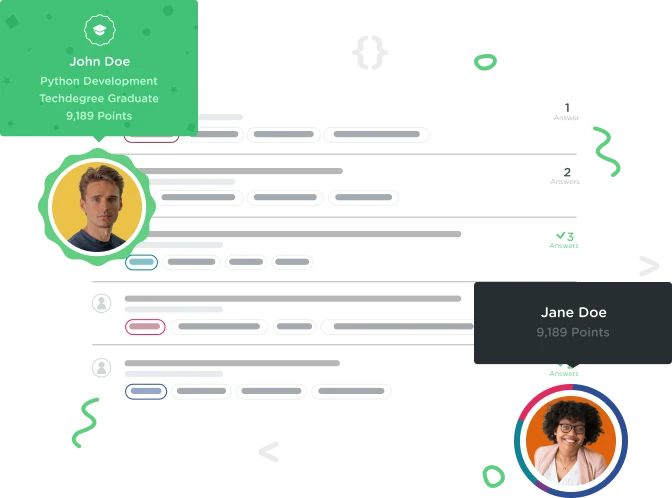

Elizabeth Collier
2,273 PointsBeneath the max function you just created, call it with two numbers and display the results in an alert dialog. Pass
Beneath the max function you just created, call it with two numbers and display the results in an alert dialog. Pass the result of the function to the alert method. For example, to display the results of the Math.random() method in an alert dialog you could type this: alert( Math.random( ) );
function max(num1, num2){
if(num1 > num2){
return num1;
} else {
return num2;
}
}
4 Answers
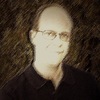
Jason Anders
Treehouse Moderator 145,860 PointsHey Elizabeth,
The second part of the challenge mainly involves calling the function you just created. If you remember, you call a function simply by typing the name with a pair of parenthesis after it. Example: functionName(); Any parameters would be passed inside the parenthesis. In this case, two numbers (of your choosing).
To complete the challenge, it is also asking that the function call be wrapped inside of an alert (which, is also a function in itself). The example given in the question is the correct syntax needed, you'd just need to change the name of the function and insert your parameters:
alert( max(4,7) );
Hope that helps and makes sense now.

Sjors Theuns
6,091 PointsHi Elizabeth,
You can display an alert with:
alert("Some text");
For this challenge you need to alert the outcome from your max function, so:
function max(num1, num2){
if(num1 > num2){
return num1;
} else {
return num2;
}
}
alert(max(72, 34));

sachin jadhav
10,052 PointsThanx....

Safwan Talha
111 Pointsfunction max (a, b) { if (a > b) { return a; } else { return b; } } alert(max(32, 56));

Ruan Davis
7,344 PointsThe code can also be written as follow, alert(Math.random(max(20,15)));
the code will start executing from the inside out. therefore the "max" function will be initiated first then math.random and lastly the alert box.