Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial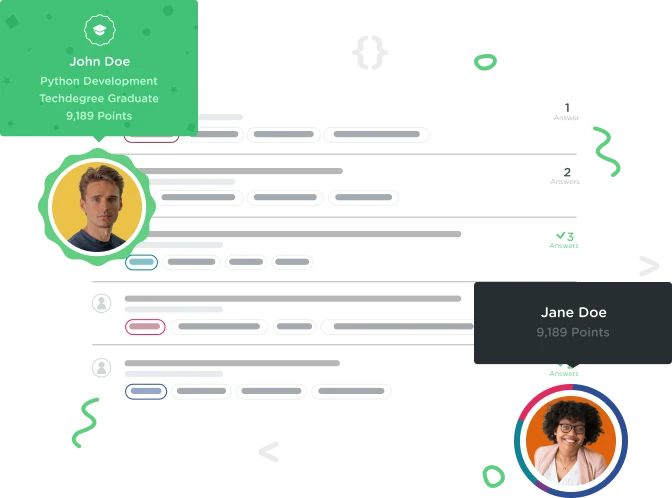
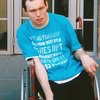
Jonny Strange
6,412 PointsBit stuck on a function question
I'm passing two arguments through the function, but I'm getting an error message saying 'the function isn't returning a number' but it is sort of. Can anyone help??
function max(number1, number2) {
if (number1 > number2) {
var largenumber = max();
}
return largenumber;
}
5 Answers

Fidel Torres
25,286 PointsHello Jonny Strange
this is your code
function max(number1, number2) {
if (number1 > number2) {
var largenumber = max();
}
return largenumber;
}
what is not right in your code
1.-*
var largenumber = max();
// this is bad practice cause this is mean to be use for recursion
// which is recall a function and execute it again
//it also most have parameters as the function requires
2.-*
if (number1 > number2) {
var largenumber = max();
}
// this is bad practice cause this only create the var largenumber if #1 > #2
//suppose you fix your code like this:
function max(number1, number2) {//max(2,8)
if (number1 > number2) {
var largenumber = number1 ;
}else{
largenumber = number2;
}
return largenumber;
//you will still have that error cause the var largenumber wasn't created
Conclutions:
function max (num1, num2){
var largenumber; //step1 --first of all create variable to store value
if (num1 > num2){ //step3 --create you code to process the info.
largenumber = num1;
} else {
largenumber = num2;
}
return largenumber; //step2 -- set the return value
}
HOPE this helps you to understand functions
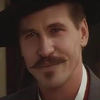
huckleberry
14,636 PointsI think what you're looking for is this...
function max(number1, number2) {
var largernumber;
if (number1 > number2) {
largernumber = number1;
return largernumber;
} else {
largernumber = number2;
return largernumber;
}
}
This will allow you to pass 2 arguments to the max function
within the function I first establish the variable largernumber before any of the other code. He'll mention it later when you get to scope and best practices but you should declare all the variables that you're gonna be using within the function, in the beginning. Even if there's no value yet, just declare the name.
Then the if statement where the two parameters within the ( ) will be compared
if number1 is greater than number2 then the condition is true and the if block will execute. It will assign the variable that we declared at the beginning of the function the value of number1 and then it will return that variable to your function call.
if number1 is less than number2 then the condition is false and the else block will execute. It will assign the variable that we declared at the beginning of the function the value of number2 and then it will return that variable to your function call.
So...
function max(number1, number2) {
var largernumber;
if (number1 > number2) {
largernumber = number1;
return largernumber;
} else {
largernumber = number2;
return largernumber;
}
}
//this will evaluate to false and will therefore assign the value of
//number2 to the variable and print that number to the console
console.log(max(17, 34));
//this will evaluate to true and will therefore assign the value of
//number1 to the variable and print that number to the console
console.log(max(24, 13));
Is that what you were looking for?
Huck -
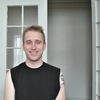
John Keener
6,808 PointsYou can't call a function inside of itself. Do you mean to assign number1 to largenumber?
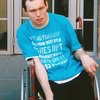
Jonny Strange
6,412 PointsNo, I want to assign the return value of the function to largenumber. Basically, I've to passing two arguments through the function, check to see which of the arguments is larger than the other and return the larger number.
function max(number1, number2) { if (number1 > number2) { return max(); } }
var largenumber = max();
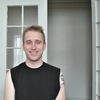
John Keener
6,808 PointsExactly what I thought. As your code is now, the function doesn't have a value because instead of assigning one to the variable that you're trying to return from it, you're assigning it the function inside of which it exists.
Try:
function max(number1, number2) {
if (number1 > number2) {
var largenumber = number1;
}
return largenumber;
}
jdh
12,334 PointsAnother solution for this Jonny Strange is below
function max (num1, num2){
if (num1 > num2){
return num1;
} else {
return num2;
}
}
As stated above, you cannot call a function within itself. In the above example, you're only getting largerNumber when number1 is larger than number2. But what about when number2 is larger than number1?
If I understand the question correctly, the above should work for you.
Jonny Strange
6,412 PointsJonny Strange
6,412 PointsThanks guys for helping me.