Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial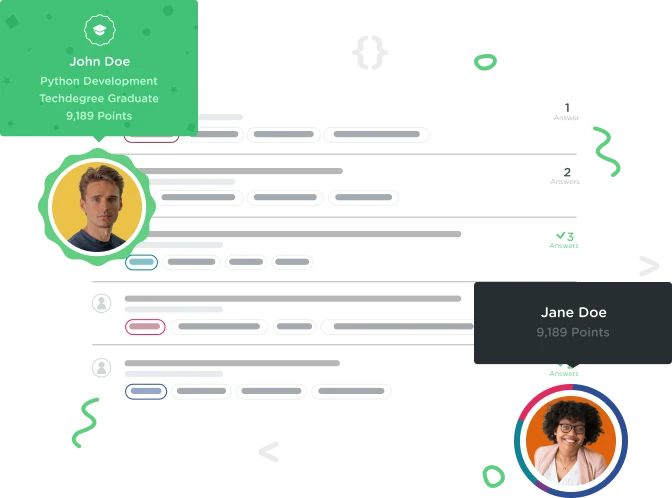
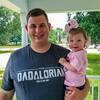
Eric Wilson
9,380 PointsBlank Console Screen - Console.WriteLine(player.SecondName); not working.
I keep getting a blank console window when I run my code using F5. I should mention that I am using a Mac and Visual Studio for Mac 2019.
I tried adding a Console.WriteLine
statement above the foreach
loop just to make sure I was checking the correct console window. I am also not getting any errors when I run the build.
What am I doing wrong?
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using Newtonsoft.Json;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResults(fileName);
fileName = Path.Combine(directory.FullName, "players.json");
var players = DeserializePlayers(fileName);
foreach(var player in players)
{
Console.WriteLine(player.SecondName);
}
}
public static string ReadFile(string fileName)
{
using(var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
public static List<GameResult> ReadSoccerResults(string fileName)
{
var soccerResults = new List<GameResult>();
using(var reader = new StreamReader(fileName))
{
string line = "";
reader.ReadLine();
while((line = reader.ReadLine()) != null)
{
var gameResult = new GameResult();
string[] values = line.Split(',');
DateTime gameDate;
if (DateTime.TryParse(values[0], out gameDate))
{
gameResult.GameDate = gameDate;
}
gameResult.TeamName = values[1];
HomeOrAway homeOrAway;
if (Enum.TryParse(values[2], out homeOrAway))
{
gameResult.HomeOrAway = homeOrAway;
}
int parseInt;
if (int.TryParse(values[3], out parseInt))
{
gameResult.Goals = parseInt;
}
if (int.TryParse(values[4], out parseInt))
{
gameResult.GoalAttempts = parseInt;
}
if (int.TryParse(values[5], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
if (int.TryParse(values[6], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
double possessionPercent;
if ( double.TryParse(values[7], out possessionPercent))
{
gameResult.PosessionPercent = possessionPercent;
}
soccerResults.Add(gameResult);
}
}
return soccerResults;
}
public static List<Player> DeserializePlayers(string fileName)
{
var players = new List<Player>();
var serializer = new JsonSerializer();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
players = serializer.Deserialize<List<Player>>(jsonReader);
}
return players;
}
}
}
Here is my Player class file:
using System;
namespace SoccerStats
{
public class RootObject
{
public Player[] Player { get; set; }
}
public class Player
{
public int Assists { get; set; }
public int BigChancesCreated { get; set; }
public int Blocks { get; set; }
public int ChanceOfPlayingNextRound { get; set; }
public int ChanceOfPlayingThisRound { get; set; }
public int CleanSheets { get; set; }
public int Clearances { get; set; }
public int Code { get; set; }
public int CostChangeEvent { get; set; }
public int CostChangeEventFall { get; set; }
public int CostChangeStart { get; set; }
public int CostChangeStartFall { get; set; }
public int Crosses { get; set; }
public int DreamteamCount { get; set; }
public int ElementType { get; set; }
public string EpNext { get; set; }
public string EpThis { get; set; }
public int ErrorsLeadingToGoal { get; set; }
public int EventPoints { get; set; }
public string FirstName { get; set; }
public string Form { get; set; }
public int GoalsConceded { get; set; }
public int GoalsScored { get; set; }
public int Id { get; set; }
public bool InDreamteam { get; set; }
public int Interceptions { get; set; }
public int KeyPasses { get; set; }
public int LoanedIn { get; set; }
public int LoanedOut { get; set; }
public int LoansIn { get; set; }
public int LoansOut { get; set; }
public int Minutes { get; set; }
public string News { get; set; }
public int NowCost { get; set; }
public int OwnGoalEarned { get; set; }
public int OwnGoals { get; set; }
public int PassCompletion { get; set; }
public int PenaltiesConceded { get; set; }
public int PenaltiesEarned { get; set; }
public int PenaltiesMissed { get; set; }
public int PenaltiesSaved { get; set; }
public string Photo { get; set; }
public string PointsPerGame { get; set; }
public string Position { get; set; }
public int Recoveries { get; set; }
public int RedCards { get; set; }
public int Saves { get; set; }
public string SecondName { get; set; }
public string SelectedByPercent { get; set; }
public int Shots { get; set; }
public bool Special { get; set; }
public string Status { get; set; }
public int Tackles { get; set; }
public int Team { get; set; }
public string TeamName { get; set; }
public int TotalPoints { get; set; }
public int TransfersIn { get; set; }
public int TransfersInEvent { get; set; }
public int TransfersOut { get; set; }
public int TransfersOutEvent { get; set; }
public string ValueForm { get; set; }
public string ValueSeason { get; set; }
public int WasFouled { get; set; }
public string WebName { get; set; }
public int YellowCards { get; set; }
}
}
1 Answer
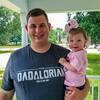
Eric Wilson
9,380 PointsAfter watching the next video, I figured out what the problem was. I don't have the Paste Special
option in my Edit Menu since I'm on Mac. So instead, I ran my JSON list through json2csharp.com to convert it into a class. It removed the underscores from the property names, and I didn't configure the JSON Deserializer to know the underscores had been removed.