Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial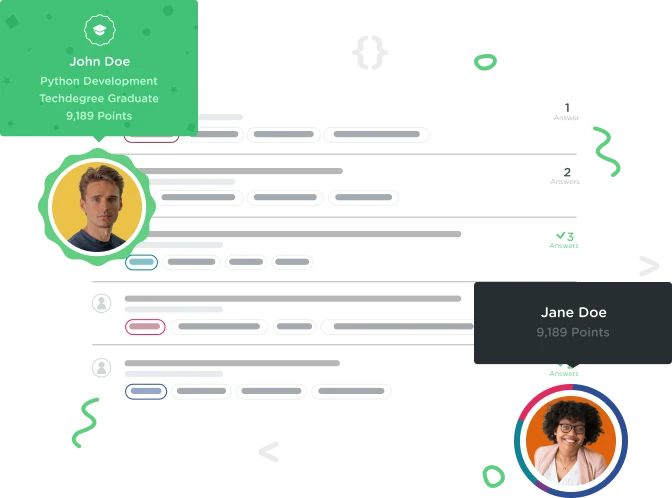

Avery Freeman-wheaton
2,273 Pointsbool charge increment - no error in preview, but cannot continue course
Hi,
I wrote a sequence to increment the charge of the GoKart battery by one (so I think). When I click on "check work" it doesn't show any errors in the preview window, but I am not allowed to move on. Here's the code:
public boolean charge() { boolean charging = false; if (!isFullyCharged()) { mBarsCount++; charging = true; } return charging; }
I have tried implementing a "while loop" to print the increment with:
while charging() { System.out.println(mBarsCount); }
Although I'm not sure if that would be correct (it gives me errors).
Anyway, any help would be appreciated. Thanks!
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean charge() {
boolean charging = false;
if (!isFullyCharged()) {
mBarsCount++;
charging = true;
}
return charging;
}
public void charged() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
public String getColor() {
return mColor;
}
}
2 Answers
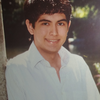
Jon Kussmann
Courses Plus Student 7,254 PointsHi Avery,
The idea behind the charge() method is you want to continuously increment mBarsCount as long as your GoKart is not fully charged. By using an if statement, you only check once if the GoKart is fully changed (and only increment it once if it is not).
A while loop works better for this, since it will run a block of code until it reaches false (in this case when the GoKart is fully charged).
I believe you were supposed to modify the given charge() method (it looks like you added a new method?).
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
I hope this helps. If not, let me know if I can clarify anything.

Hayes McCardell II
643 PointsThe exercise isn't asking you to print the output in the while loop, it wants you to actually implement the increment inside the while loop.
public void charge() {
while (!isFullyCharged()){
mBarsCount++;
}
}
I'd suggest being careful when you change return types on methods and/or insert new variables that haven't been specified, as well.
There are two beasts to tackle here. One is the language itself, and the other is the specific requirements that the exercise is checking for. If you crank up your own IDE and fiddle with the code, some solutions might work perfectly. But if you try to put that more elegant and robust code into the exercise, it may fail because it's just seeing things it's not expecting.

Avery Freeman-wheaton
2,273 PointsHi,
yes, tinkering is my nature so it's hard for me to ignore wanting to fiddle with IDEs (I set up Eclipse long before I started with TreeHouse and struggled with poor-quality tutorials on Youtube).
However, for the purposes of these exercises I am simply using workspaces in an attempt to circumvent the pitfalls of getting overly involved in the infrastructure-side of coding.
Thanks for your input, I really appreciate it.
Avery Freeman-wheaton
2,273 PointsAvery Freeman-wheaton
2,273 PointsHi,
Yes, this was helpful (as was the other answer, thank you!).
The example shown in the video had the while loop occurring in a different class, so it was a little confusing to me.
Here's how I implemented the code:
Thanks again!