Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial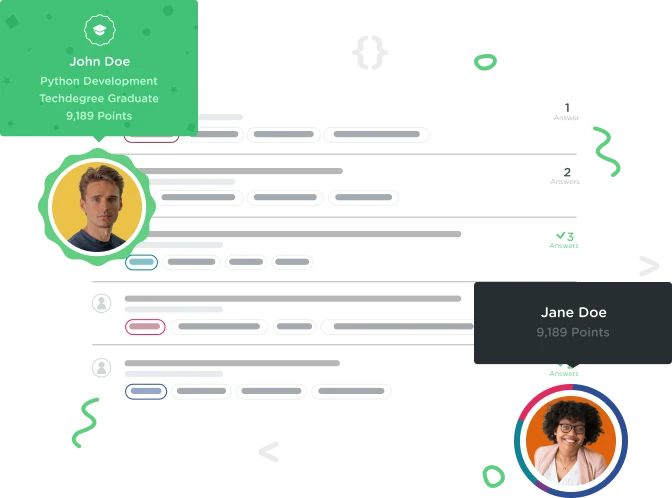

Nicolas Hémidy
8,481 PointsBranch in ruby
Hello everyone !
I have question regarding branch in ruby. I'm trying to create a quiz in ruby, here is the code :
def get_name ()
print "Please tell me your name : "
return gets.chomp
end
def welcome (name)
puts "Welcome #{name}!"
if (name == "Julie")
puts "What a lovely name!"
end
end
def get_answer ()
print "Do you want to try a little quiz ? (yes/no): "
return gets.chomp
end
def quiz (answer)
if (answer == "yes") || (answer == "Yes")
puts "Let's go !"
elsif (answer == "No") || (answer == "no")
puts "Ok :("
else
puts "Sorry I did not understand.."
end
end
def get_answer1 ()
print "Here is the first question !"
print " What is my best french movie ?: "
return gets.chomp
end
def question1 (answer1)
if (answer1 == "Le premier jour du reste de ta vie") || (answer1 == "le premier jour du reste de ta vie")
puts "That's my girl !"
else
puts "Are you serious ??"
end
end
name = get_name()
welcome (name)
answer = get_answer()
quiz (answer)
answer1 = get_answer1()
question1 (answer1)
I would like to know how i build different branch in ruby ? To be more specific, how can i create a different path if an answer is yes or no. Do i need to create this specific path inside the def question1(answer1) ?
I really thank you in advance, Nicolas
4 Answers

Daniel Cunningham
21,109 PointsStart by separating your questions from the procedure to gather the answer. get_name, get_answer, and get_answer1 are effectively the same method, so separate the 'answer' from the question entirely. In fact, rather than making a method for each question, you should consider storing the questions into variables and calling them into a single 'question' method. <br>
Consider creating a variable with all of the questions within a single array (questionvar = [q1,q2,q3]), put all of the questions inside, and do the same with the answers (answers =[answer1,answer2,answer3]) you might be looking for to specific questions. If there are specific results you want to acheive for each answer (result= ["correct","wrong!"]), you could build a variable for that as well. <br> An example question design might include: <br> <br>
def question(questionvar[x], answervar,resultsvar[x],resultsvar[y])
puts questionvar1
answer = gets.chomp
if answervar.include? answer
resultvar[x]
else
resultvar[y]
end
end
<br> Keeping the format simple for asking the questions can give you a lot more flexibility in producing new branches... and it will save you a lot of redundant coding.

Nicolas Hémidy
8,481 PointsHi Daniel,
I just started the course about arrays this morning and i understand your answer. I will work on this solution.
I really thank you for your answer, Nicolas

Nicolas Hémidy
8,481 PointsHello Daniel,
If you have 5 min, no hurry :) , I tried this code :
q1 = "Please tell me your name : " q2 = "Do you want to try a little quiz ? (yes/no): " q3 = "Here is my first question! What is my best french movie?: " r1 = "What a lovely name !" r2 = "Ok, nice to meet you #{answer}! " r3 = "Correct!" r4 = "Brilliant!" r5 = "Wrong.." a1 = "Julie"
questionvar = [q1,q2,q3] resultvar = [r1,r2,r3,r4,r5] answervar = [a1,a2,a3]
def question(questionvar[1], answervar[1], resultvar[1], resultvar[2]) puts questionvar[0] answer == gets.chomp.downcase if answervar[1].include? answer resultvar[1] else resultvar[2] end end
Unfortunatly, it produces this error :
array_training.rb:15: syntax error, unexpected '[' expecting ')' def question(questionvar[1], answervar[1], resultvar[1...
Could you please explain what I did wrong ?
Really thank you in advance, Nicolas

Daniel Cunningham
21,109 PointsYou have to first define the method. After you define it, then you can call it. it looks like you're attempting to do both while defining the method. <br>
def question(question,answer,result1,result2)
answeruser = gets.chomp.downcase
if answer.include? answeruser
result1
else
result2
end
end
#after that, call the question method
question(questionvar[1], answervar[1], resultvar[1], resultvar[2])
Just in case, Do NOT forget the Arrays start at 0. So if you want the very first element of array questionvar, you need to call questionvar[0].
Good luck!

Nicolas Hémidy
8,481 PointsThank you for your help Daniel !