Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial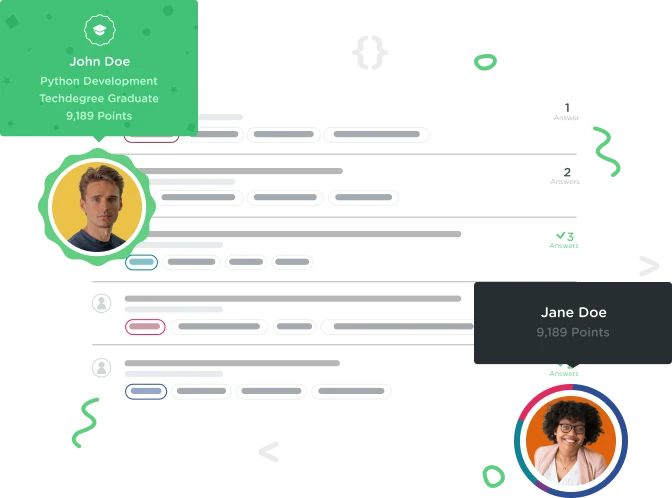
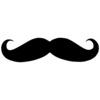
hum4n01d
25,493 PointsBrowser sync with Gulp or other live reload method
I am trying to follow this tutorial to reload the browser whenever I change my jade/pug files but it isn’t working. Any ideas? Here is my code:
var gulp = require('gulp'),
pug = require('gulp-pug')
browserSync = require('browser-sync').create(),
reload = browserSync.reload;
gulp.task('pug', function() {
return gulp.src('**.jade')
.pipe(pug())
.pipe(gulp.dest('./'));
});
gulp.task('watchPug', function() {
gulp.watch('**.jade', ['pug']);
});
// Static server
gulp.task('serve', function() {
browserSync.init({
server: {
baseDir: './'
}
});
gulp.watch('**.html').on('change', reload);
});
gulp.task('default', ['watchPug']);
If there is a better way to reload my browser whenever I change a file, please let me know.
4 Answers
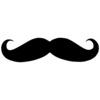
hum4n01d
25,493 PointsI found this and it works perfectly.
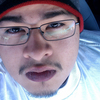
Chyno Deluxe
16,936 Pointsyou are missing a comma after pug require. Im not sure if that will fix the problem but most likely if everything else is correct this would prevent it from working.
var gulp = require('gulp'),
pug = require('gulp-pug'), //you were missing a comma here
browserSync = require('browser-sync').create(),
reload = browserSync.reload;
gulp.task('pug', function() {
return gulp.src('**.jade')
.pipe(pug())
.pipe(gulp.dest('./'));
});
gulp.task('watchPug', function() {
gulp.watch('**.jade', ['pug']);
});
// Static server
gulp.task('serve', function() {
browserSync.init({
server: {
baseDir: './'
}
});
gulp.watch('**.html').on('change', reload);
});
gulp.task('default', ['watchPug']);
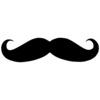
hum4n01d
25,493 PointsI fixed the comma, but it didn’t solve the problem
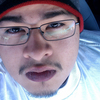
Chyno Deluxe
16,936 PointsI don't use jade so I'm not sure If the following code will work but you can try this. This follows my current set up with the exception that I use a CSS preprocessor (SCSS) rather than an HTML preprocessor.
var gulp = require('gulp'),
pug = require('gulp-pug'),
browserSync = require('browser-sync').create(),
reload = browserSync.reload;
gulp.task('pug', function() {
return gulp.src('*.jade')
.pipe(pug())
.pipe(gulp.dest('./');
});
// Static server
gulp.task('serve', ['pug'],function() {
browserSync.init({
server: './'
});
gulp.watch('*.jade', ['pug']);
gulp.watch('*.html').on('change', reload);
});
gulp.task('default', ['serve']);
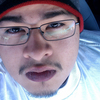
Chyno Deluxe
16,936 PointsI just realized that you weren't running the serve gulp task. That is why browser-sync was not running. You need to add it to your defualt task.
gulp.task('default', ['serve', 'watchPug']);
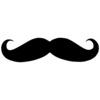
hum4n01d
25,493 PointsThanks, I found this and it works perfectly.