Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial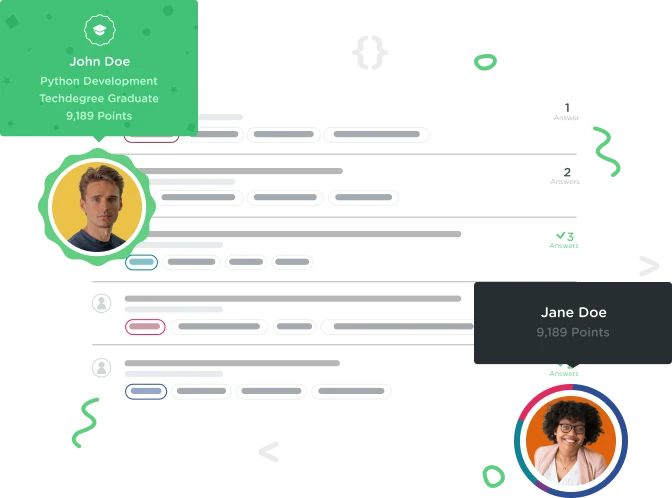
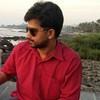
Ravi Yadav
10,152 PointsBuild a basic PHP website Code Challenge Task 4 of 4
PalprimeChecker objects have a method called isPalprime(). This method does not receive any arguments. It returns true if the number property contains a palprime, and it returns false if the number property does not contain a palprime. (Tip: 17 is not a palprime.) Turn the second echo statement into a conditional that displays "is" or "is not" appropriately. The conditional should call the isPalprime method of the $checker object. If the isPalprime method returns true, then echo "is"; otherwise, echo "is not".
My answer to this is
<?php
include('class.palprimechecker.php');
$checker = new PalprimeChecker;
$checker->number = 17;
echo "The number " . $checker->number . ' ';
if ($checker->isPalprime()) {
echo "is";
} else {
echo "is not";
}
?>
But I get an error saying
Bummer! It seems like you are calling the right method and you have the removed various placeholder characters (##|()), but something in the output is not quite right. Please double-check the preview.
Can someone help me with what exactly is expected here?
3 Answers
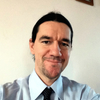
Alberto Martinez Pascual
6,714 PointsHello Ravi, the errors are sometimes not very precise. Perhaps is it only that you should put another echo after closing the else?:
echo "The number " . $checker->number . ' ';
if ($checker->isPalprime()) {
echo "is";
} else {
echo "is not";
}
echo " a Palprime";
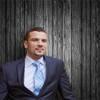
Andrew Dovganyuk
10,633 PointsTry this it should work!
include("class.palprimechecker.php");
$checker = new PalprimeChecker();
$checker->number = 17;
if($checker -> isPalprime()){
echo "The number " . $checker->number . " is a palprime.";
} else {
echo "The number " . $checker->number . " is not a palprime.";
}
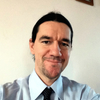
Alberto Martinez Pascual
6,714 PointsThanks Ravi! ItΒ΄s done!
Ravi Yadav
10,152 PointsRavi Yadav
10,152 PointsThank you, It did work. I tried your method and it worked. Can you please edit your answer with a period after Palprime in the last echo. Otherwise it still gives the same error. Thank you.