Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial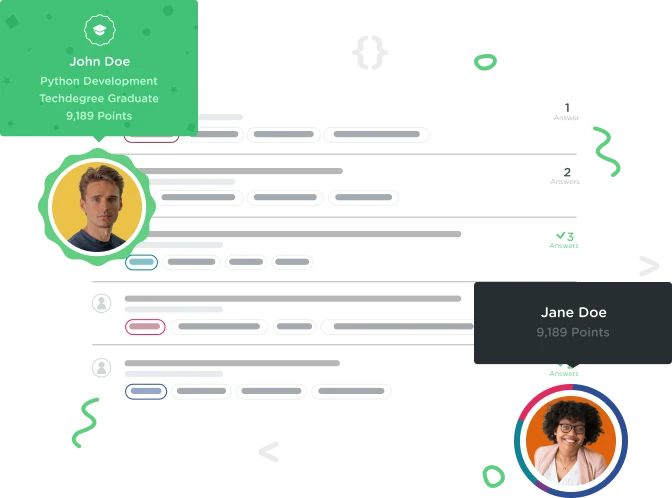

raviteja chintala
Courses Plus Student 32 PointsBuild a simple app that draws and listens for taps
Requirements:
1) You will have a main Activity. It will have one specialized view called
MyDrawView that extends View and implements OnClickListener,
OnLongClickListener, and OnTouchListener.
a. It should be at least 300 by 400.
b. It will have a private ArrayList (or two depending on whether you
store points or x,y values) to hold the x and y values for points for a
figure that will be drawn.
c. It will have a private Boolean drawFigure that is true when we should
draw the figure. If it is false, we will just draw the points that we
currently have.
d. It will have two private float values: lastx, lasty where we will record
the last position of a touch down.
e. You will override the onDraw method. You will need to create a scene
(be creative) using all of the following draw methods for a canvas:
i. drawArc
ii. drawRect
iii. drawOval
iv. drawText
v. Depending on the value of drawFigure, you will do one of two
things. If it is true, you will use drawLine between every pair
of adjacent points in the list to draw the appropriate closed
figure. If it is false, use drawPoint on each of the x,y pairs. In
both cases, use a stroke of width 5.0 and color MAGENTA.
f. You must change the pen so that your drawing uses
i. At least three different colors
ii. At least two different widths
iii. A figure that is stroked and a figure that is filled.
g. In OnClick you will check drawFigure. If it is true, clear the list(s) of
x,y values and set drawFigure to false. In all cases, add lastx and lasty
from the last touch to the lists of values.
h. OnLongClick, add lastx and lasty to the list of values and then add the
first point in the list(s) to the end of the list(s). Set drawFigure to
true.
i. OnTouch you will check to see if the MotionEvent is TOUCH_DOWN, if
so, set lastX to event.getX() and similarly for y. Then return the super
call on the method. (We do this because the click listener does not get
an event that notes the location of the click, just that a click happened
in the view. We could do everything in onTouch, but then we have to
write the logic for click/longclick ourselves.)
2) The app should be tested properly and should not crash in any scenario. Use
appropriate Toast messages