Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial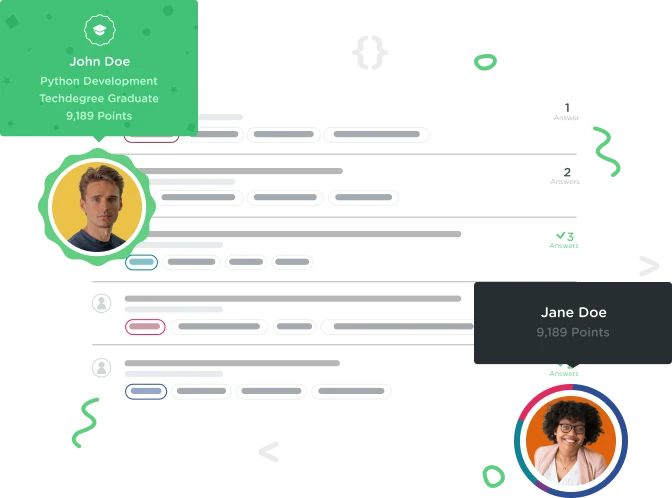

Natenda Manyau
3,005 PointsBummer! Hmmm, remember that the indexOf method on strings returns -1 if the char is missing, or greater than that if it
am not too sure what is wrong now. please help
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
boolean hand = mHand.indexOf(tile) >= 0;
if (hand) {
mHand += tile;
}else {mHand.indexOf(tile) <= 0;}
return false;
}
}
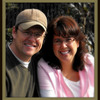
Kevin McFarland
5,122 PointsMaybe you've already resolved your problem... If not see comments below code.
public boolean hasTile(char tile) {
boolean hand = mHand.indexOf(tile) >= 0;
if (hand) { 2
mHand += tile;
} else { mHand.indexOf(tile) <= 0;} <------------
return false;
}
See the <-------- above: curly braces are isolating "return false" from the if/else statement - so it will always return false. See your other curly braces. The "return false" needs to be within the scope {} of the else statement.
2 Answers

Dan Johnson
40,533 PointsThe hasTile method's responsibility is just to check if a tile exists, so no need to modify mHand here.
Now when checking for the occurrence of the tile, you'll want to be looking specifically for -1 if it doesn't contain it (0 is a valid index that could contain the tile):
public boolean hasTile(char tile) {
if(mHand.indexOf(tile) == -1)
return false;
else
return true;
}
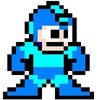
Robert Richey
Courses Plus Student 16,352 PointsHi Natasha,
Your code will run if you remove the conditional from the else statement - which, cannot have a conditional statement -- and -- if you put a return true;
statement inside the if block. Only if
and else if
may have conditional statements.
After that, it's a matter of personal preference how you implement a solution. This will hopefully give you the sense that there are many different ways of writing code to solve a problem. Here is another example solution not yet mentioned.
public boolean hasTile(char tile) {
if(mHand.indexOf(tile) >= 0) { return true; }
return false;
}
Notice I didn't bother with an else clause, because if the if
statement evaluates to false, code will continue executing after the if
block - and in this case will execute the next line return false;
Finally, here is a one line solution using the ternary operator ?:
, just for fun.
public boolean hasTile(char tile) {
return (mHand.indexOf(tile) >= 0) ? true : false;
}
Kind Regards
MUZ140058 Wellington Bore
8,908 PointsMUZ140058 Wellington Bore
8,908 PointsIn your if conditional, that is where you need to reference (mHand.indexOf(tile)>=0) and this should return true.hope the code below will help you past the code challenge
} }