Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial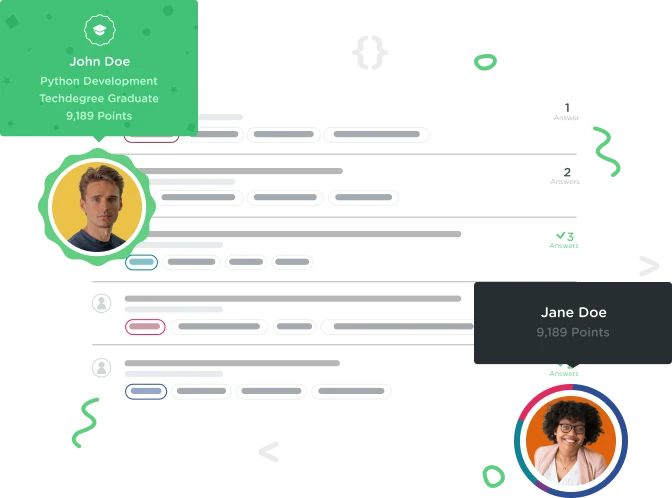

Eric Hertenstein
7,053 PointsBut I AM throwing the exception before changing the mBarsCount field! Aren't I?
Here's my version of the drive function, as requested:
public void drive(int laps) {
// Other driving code omitted for clarity purposes
int newBars = mBarsCount -= laps;
if (newBars < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount = newBars;
}
What am I doing wrong here? Is it bugs? Have we got bugs?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
int newBars = mBarsCount -= laps;
if (newBars < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount = newBars;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
4 Answers
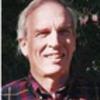
jcorum
71,830 PointsFirst check to see if mBarsCount - laps is less than 0, and if it is throw the exception. Otherwise, decrement the Battery mBarsCount -= laps:
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (mBarsCount - laps < 0) {
throw new IllegalArgumentException();
} else {
mBarsCount -= laps;
}
}

Derek Markman
16,291 PointsThe challenge instructions are: Okay, so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains".
So all we need to check for is if the requested number of laps (the int argument passed into your overloaded drive method) is greater than or less than the current number of energy bars available(mBarsCount).
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if(laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
} else {
mBarsCount -= laps;
}
}
What's happening is, when the overloaded drive method that takes an int parameter is invoked, like in the drive method above it, only if the number of laps that's passed in to the drive method's argument is greater than mBarsCount then it will throw an IllegalArgumentException, otherwise, mBarsCount = mBarsCount - laps will occur and no exception will be thrown.
Let me know if you have any other questions.

Eric Hertenstein
7,053 PointsThanks guys. Both of your methods are definitely valid — Derek, yours works, but only throws the exception if the gokart is at MAX_BARS. jcorum, your extra math will throw the exception even if it's say, half full, so that fits the bill.
Thing is, I was using a type of method like in the lesson before about pez dispensers:
- creating a new temporary int variable for the post-driving state of the battery
- if that's negative, throwing the exception
- if not, setting the mBarsCount equal to the aforementioned temporary variable.
But the test was all, "Bummer! don't forget to throw the exception before you change the variable!" But isn't my method a valid way to do that? Why was I getting the error?
public void drive(int laps) {
int newBars = mBarsCount -= laps;
if (newBars < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount = newBars;
}

Derek Markman
16,291 PointsTry to run that code again except assign newBars to mBarsCount - laps. Not -=. See if that works.
int newBars = mBarsCount - laps;

Eric Hertenstein
7,053 PointsThat works; I new it was something simple. Thanks Derek!

Derek Markman
16,291 PointsNo problem.