Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial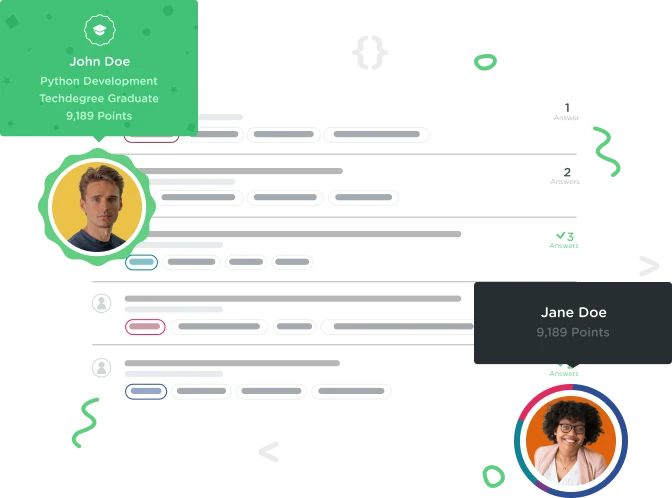

terence lara
693 PointsC# Basic final part 2
So when I ran this in Visual Studio the count variable is not working. The console ask for a value and if a number larger than 0 is entered, "Yay!" is printed but only once. The program is not running through the loop until the count variable and user input are the same. Thanks for the help in advance.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var count = 0;
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\" : ");
var input = Console.ReadLine();
try
{
var inputTimes = int.Parse(input);
if (inputTimes <= count)
{
Console.WriteLine("That is not enough for A Yay!");
continue;
}
else
{
Console.Write("Yay!");
count++;
}
}
catch (FormatException)
{
Console.WriteLine("That is invalid input. Enter a whole number.");
continue;
}
}
}
}
}
1 Answer
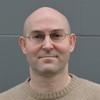
Ted Dunn
43,783 PointsYou never created the loop necessary to print the word "Yay!" more than once. Instead of the else clause, you can do the following:
for(int i = 1; i <= inputTimes; i++)
{
Console.WriteLine("Yay!");
}
terence lara
693 Pointsterence lara
693 PointsThank you. I got it to work using the for loop. I put a 1 in place of the i for the condition i <= inputTimes at first and my program just produced a bunch of Yays.