Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial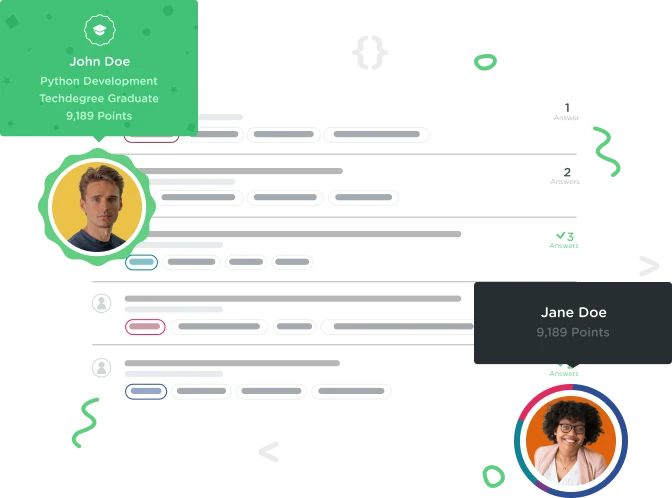
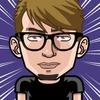
Konstantinos Gialantzis
677 PointsC# Basics: Bonus Exercises (Arithmetic Calculator and Averager)
This is my homework about Arithmetic Calculator and Averager. I know the code is a little complicated, but i want your opinions about.
Averager:
using System;
namespace Treehouse.Averager
{
class Program
{
static void Main(string[] args)
{
var runningTotal = 0.0;
var countEntries = 0;
while (true)
{
Console.Write("Enter a number or type \"done\" to see the average (or type \"quit\" to exit): ");
var entry = Console.ReadLine();
if (entry.ToLower() == "done")
{
Console.WriteLine("Average = " + runningTotal / (double)countEntries + " (Enter another number to add it to your average)");
}
else if (entry.ToLower() == "quit")
{
break;
}
else
{
try
{
var number = double.Parse(entry);
runningTotal += number;
countEntries++;
}
catch (Exception e) when (e is FormatException || e is OverflowException || e is DivideByZeroException)
{
Console.WriteLine("Please type a valid numeric value");
continue;
}
}
//Console.WriteLine(countEntries); //ο συνολικός αριθμός εισόδων αριθμών από τον χρήστη για τον υπολογισμό του μέσου όρου
}
}
}
}
Arithmetic Calculator:
using System;
namespace Arithmetic_Calculator
{
class Program
{
static void Main(string[] args)
{
string entry;
string operatorSymbol;
double nu1;
double nu2;
while (true)
{
Console.Write("Enter a number (or type \"quit\" to exit): ");
entry = Console.ReadLine();
if (!double.TryParse(entry, out nu1) && entry.ToLower() != "quit")
{
Console.WriteLine("Please type a number or \"quit\"", entry);
continue;
}
if (entry.ToLower() != "quit")
{
nu1 = double.Parse(entry);
Console.Write("Enter a math operator (or type \"quit\" to exit): ");
operatorSymbol = Console.ReadLine();
if (operatorSymbol.ToLower() == "quit")
{
break;
}
while(true)
{
if (operatorSymbol != "*" && operatorSymbol != "-" && operatorSymbol != "+" && operatorSymbol != "/" && operatorSymbol != "%" && operatorSymbol !="^")
{
Console.Write("Please type a valid math operator: ");
operatorSymbol = Console.ReadLine();
}
else
{
break;
}
}
Console.Write("Enter another number (or type \"quit\" to exit): ");
entry = Console.ReadLine();
if (!double.TryParse(entry, out nu2) && entry.ToLower() != "quit")
{
Console.WriteLine("Please type a number", entry);
continue;
}
if (entry.ToLower() == "quit")
{
break;
}
nu2 = double.Parse(entry);
if (operatorSymbol == "+")
{
Console.WriteLine("Result is: " + (nu1 + nu2));
}
else if (operatorSymbol == "-")
{
Console.WriteLine("Result is: " + (nu1 - nu2));
}
else if (operatorSymbol == "*")
{
Console.WriteLine("Result is: " + (nu1 * nu2));
}
else if (operatorSymbol == "/")
{
Console.WriteLine("Result is: " + (nu1 / nu2));
}
else if (operatorSymbol == "%")
{
Console.WriteLine("Result is: " + (nu1 % nu2));
}
else if (operatorSymbol == "^")
{
Console.WriteLine("Result is: " + Math.Pow(nu1, nu2));
}
}
else
{
break;
}
}
}
}
}
1 Answer

Erick R
5,293 PointsI like it! Here are some tips for you my friend. You explicitly declared the types in the Arithmetic calculator. However, you used the keyword var for the Averager. Although there is no problem executing the code, some people might not know what the type of the variable is even if its already assigned. Use var when it is known explicitly and locally for example in a counter for( var i = 0; ....; i++). Also, consider using the string.Format method to create a structure where you can replace the operators. That way you would just switch on the type of operator. There are various ways to skin a cat. You already made a successful Averager and Arithmetic. Why not create an abstract class that defines the structure of the Arithmetic class and hook it up to a interface. That way, Averager can implement the Interface as well :D. Consider using Enumerators for operators. Throw in some computed properties. Heck make it multi-thread! As long as you are happy with it, it works, and you have fun that's all there is to it.
Konstantinos Gialantzis
677 PointsKonstantinos Gialantzis
677 PointsThank you for your answer Erick! I care to make the code much better! :D