Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial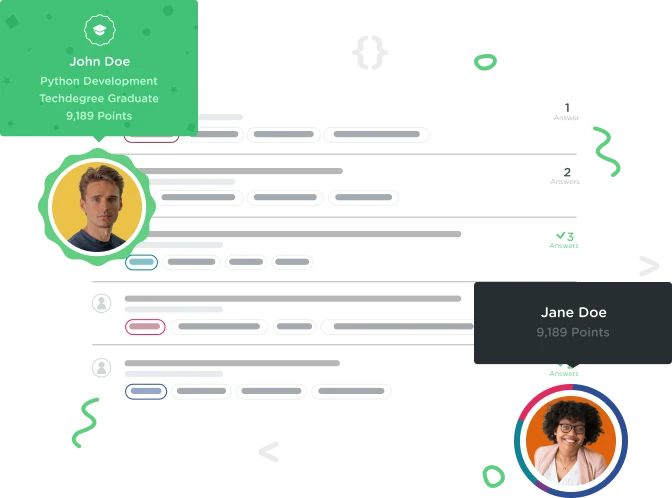
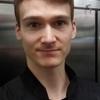
lukeoberrieder3
2,261 PointsC# Basics Code Challenge: Final - Task 2 of 3 - "Bummer! Your code took too long!"
When I run this code in visual basic, everything is fine. But when I copy and paste it to the compiler in Treehouse, the code doesn't run and I get a message saying it took too long to run. This problem started when I had to catch an ArgumentNullException.
My question is, how do I make the program faster or fix the underlying issue I'm not seeing? Feel free to ask questions. Thanks for any help!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var yayRunningTotal = 0;
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var yayLimit = int.Parse(entry);
while (yayRunningTotal < yayLimit)
{
yayRunningTotal += 1;
Console.WriteLine("Yay!");
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (ArgumentNullException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
1 Answer

andren
28,558 PointsThere is nothing wrong with your code, in fact it's quite through, but that is part of the problem. Challenges on Treehouse are often designed with very specific solutions in mind, and only designed with certain features in mind. The code you have written does more than what the challenge expects it to do, and thus it breaks the code checker.
Using a while loop to reprompt a user for an answer when they input something invalid makes perfect sense, but it is not something the task instructed you to do, and it is in fact not something the task expects you to do. The code checker that verifies the code for this challenge is only setup to provide input to the method once. Prompting it for input multiple times will lead to the code being rejected.
When the instructions asked you to catch the FormatException
and print out a message that is in fact all they expected you to do, nothing beyond that. So if you remove all of your looping and just keep the exception handling like this:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var yayRunningTotal = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var yayLimit = int.Parse(entry);
while (yayRunningTotal < yayLimit)
{
yayRunningTotal += 1;
Console.WriteLine("Yay!");
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
Then your code will be accepted. Keep in mind that the same things applies to task 3. Task 3 also involve adding some input validation, but there also you are not meant to do anything beyond printing a message.
And again, don't take this as a fault in your code or coding skills, your solution is actually very good. It's just one the challenge is not setup to handle.
lukeoberrieder3
2,261 Pointslukeoberrieder3
2,261 PointsI see. Got it fixed. Thanks!