Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial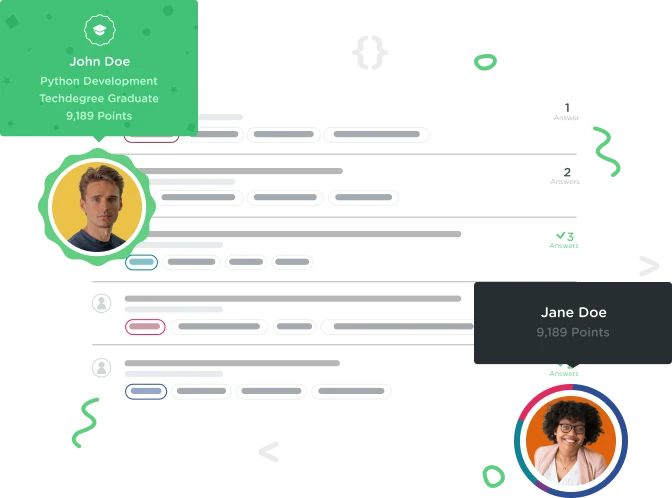

Nick Torchio
912 PointsC# Basics Final 1/3
Hey everybody. I've been working on my C# Basics Final and would like some help. I was making great progress until the error codes came back as "Bummer! Try again!". I see this as slightly good news though, because I think I'm really close. Prior to this my errors were that the code would run, but the user would enter 5 and it would only print 1 time. When I was getting that error I changed my If loop into a While loop. I know that adding a "continue;" line to a loop will make that loop restart, but does that recheck the parameters I have within the ()? Any guidance would be greatly appreciated and while I may be close I would also love for somebody to show me an example of how to solve this prompt differently just so I can see another way to get to the same result. Thanks TTH Community.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
int number = int.Parse(input);
int counter = 0;
while (counter != number)
{
Console.WriteLine("\"Yay!\"");
continue;
}
counter = counter + 1;
}
}
}
1 Answer

andren
28,558 PointsYour loop doesn't exactly follow the intended approach to this task, but it is quite close and will indeed work with just a tiny adjustment.
First I'll address some of your questions:
As you state loops do restart (start from the top) when you use the continue
statement, however loops also automatically restart once they have gone though all of the code in the code block. Meaning that placing a continue
statement on the last line is entirely redundant, as the loop would have reset either way. The continue
statement is meant as a way to restart a loop earlier than it normally would, it's not meant to be used at the end of it.
Also as a somewhat nitpciky but also somewhat important aside, there is no such thing as an "if loop", if
statements are conditional statements that either run if a condition is fulfilled or don't run. They don't run the code multiple times, which is what the term loop refers to in programming.
Anyway getting back to the meat of your question, the reason why your code is currently failing is that you set the condition of you loop to be that the counter should not be equal to the input number, but then you don't actually change the counter within the loop. Remember that as long as the looping condition is true the loop continues to run the same code over and over again. Since the condition never becomes false the loop never ends which means that your code will print out the message indefinitely.
This can be solved by simply moving your counter = counter + 1;
line to be within the loop, currently it is directly below and therefore outside the loop, that is the core issue with your solution.
Basically remove your continue
statement and move the counter increasing code to the place where the continue
used to be and your code should work fine.
Nick Torchio
912 PointsNick Torchio
912 PointsThank you for the explanation about the continue statement and the If loops. I really appreciate those clarifications.
Funny that it was that simple. When I had it previously set as an If function I had the counter line within the block and I got a different error which ultimately led me to moving it outside the block. I hadn't thought to try moving it back since I changed it back to a While loop.
Once again, thank you so much for the very detailed explanations for me.