Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial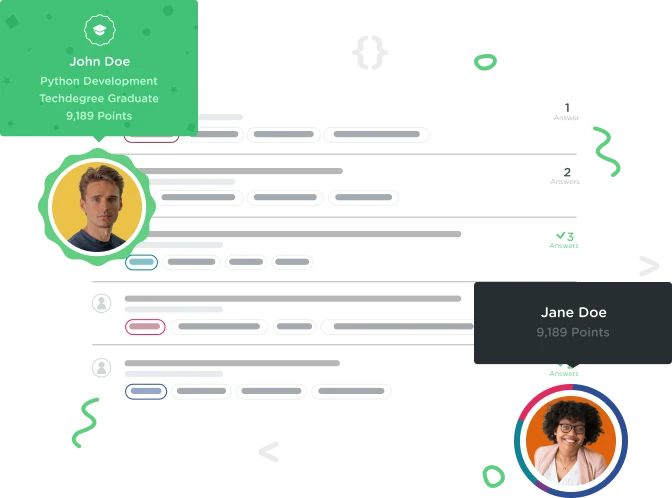

Andrew Perry
5,266 PointsC# basics final challenge task 2/3
When I enter this code in workspaces it works but I keep getting the message " oops it looks like the first challenge is no longer passing"
Here is the question: Add input validation to your program by printing “You must enter a whole number.” if the user enters a decimal or something that isn’t a number. Hint: Catch the FormatException.
Can someone please tell me what is wrong with my code?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
var redo = int.Parse(input);
try
{
while (redo >0)
{
Console.WriteLine("Yay!");
redo -=1;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number!");
continue;
}
}
}
}
}
5 Answers
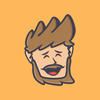
Sam Baines
4,315 PointsHi Andrew - it quite a challenge this one, mainly because you need to realise that the input is only required to be asked once (which is not clearly stated but thats a different issue) - so you dont need the input to be in the while loop.
I recently passed this challenge with the following code:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var entry = 0;
try {
Console.Write("Enter the number of times to print \"Yay!\": ");
var entryParsed = int.Parse(Console.ReadLine());
if (entryParsed < 0) {
Console.Write("You must enter a positive number.");
}
else
{
entry += entryParsed;
}
}
catch (FormatException)
{
Console.Write("You must enter a whole number.");
}
var x = 0;
while (true) {
if (x < entry) {
Console.Write("Yay!");
x++;
}
else
{
break;
}
}
}
}
}

william williams
2,912 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
int counter = 0;
try
{
var total = int.Parse(entry);
while (total > counter)
{
Console.WriteLine("Yay!");
counter +=1;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number");
}
Console.ReadLine();
}
}
}

Patrick Wan
685 Pointsusing System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
int entry = Int32.Parse(Console.ReadLine());
for(int i = 0; i < entry; i++)
Console.WriteLine("Yay!");
}
catch(FormatException)
{
Console.Write("You must enter a whole number.");
}
}
}
}

Nicholas Wallen
12,278 Pointsusing System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": "); var entry = Console.ReadLine();
try
{
int num = int.Parse(entry);
for (int i=0; i<num; i++)
System.Console.WriteLine("\"Yay!\"");
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}

kyle miles
Full Stack JavaScript Techdegree Student 458 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
// Checks for input error...
try
{
int count = int.Parse(input);
if( input > 0 )
{
Console.WriteLine("You must enter a positive number.");
}
else
{
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
}
// Catches FormatException Error
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}