Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial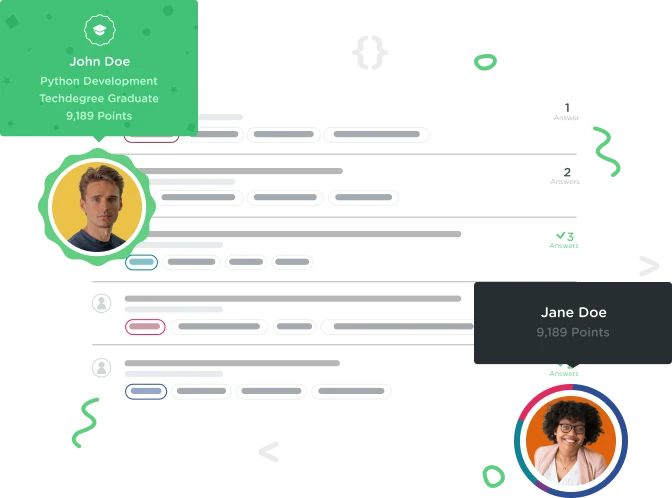
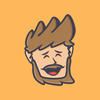
Sam Baines
4,315 PointsC# Basics Final Code Challenge - is there a better code answer?!
Hi - I've completed the final code challenge on the C# Basics course with the following code - but I want to know from someone more experienced if there is a better way to achieve the same result? Or if my code can be refactored better?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var entry = 0;
try {
Console.Write("Enter the number of times to print \"Yay!\": ");
var entryParsed = int.Parse(Console.ReadLine());
if (entryParsed < 0) {
Console.Write("You must enter a positive number.");
}
else
{
entry += entryParsed;
}
}
catch (FormatException)
{
Console.Write("You must enter a whole number.");
}
var x = 0;
while (true) {
if (x < entry) {
Console.Write("Yay!");
x++;
}
else
{
break;
}
}
}
}
}
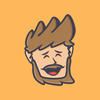
Sam Baines
4,315 PointsHi Hendrik and thanks for the response - that makes a lot of sense (I'm sure I tried to put the conditional statement in the while loop but for some reason the challenge didn't accept it?!) - but the code you posted actually needs altering a bit now that I have looked at the MSDN for the while loop. EDIT: I SEE YOU ADDED THE INCREMENT NOW. But at least I learnt you can add the increment into the conditional directly according to the MSDN.
To make the code better it should be:
while (x++ < (entry + 1))
{
Console.Write("Yay!");
}
The conditional has to have an increment in it hence the (x++) otherwise the while loop in your code would run continuously as the var x would never change and thus would always be less than var entry. But the (x++) increment stops this. Secondly I am not sure if I can put entry + 1 in the conditional like that or if I would have to add it outside the loop to the entry variable (If someone could clear that up it would be helpful?) but if I can then yes the loop without the break would be better. Less code, easier to read etc.
3 Answers

Hendrik Heim
1,012 PointsYou can add <var> +1 in a loop condition.
Edit: instead try
While(x++<=entry)
Shortest version i guess
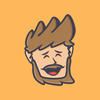
Sam Baines
4,315 PointsYeah - I thought I can either add the +1 to the entry variable when I add the entryParsed variable to it earlier on in the code to make sure it prints the correct amount of 'Yay!' based on using just the less than '<' operator - again I tried the '<=' operator in the while condition and it didn't pass the challenge so that's what probably threw me on that bit. But good advice nonetheless.

Hendrik Heim
1,012 PointsYeah, I find it hard too, to find matching Code, regarding their expectation.
There are many ways to solve a problem, however a "real" compiler would be great. :-)

Filip Ek
1,296 PointsKinda frustrating. Been trying for 45 minutes now on step 3 but it dosen't work. Not showing any errors and it works fine in Visual Studio. Took 5 minutes to write the code, so have been wasting 40 minutes on nothing. So I just copied your code and it passed, so thank you.
But I love the video tutorials, great explaining :)
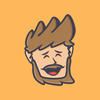
Sam Baines
4,315 PointsYeah the code challenges are very specific so just because it passes in Visual Studio doesn't mean it meets the criteria to pass the challenge - but if it works in Visual Studio at least you know your code will work in a real world scenario. Make sure you understand why this code passes the challenge though otherwise these basics might give you issues in the future. Also remember the MSDN is you friend.
Hendrik Heim
1,012 PointsHendrik Heim
1,012 PointsI'd change:
to
What you are doing is constructing an endless loop and break if your condition fails. Why not building a loop on this condition?;)