Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial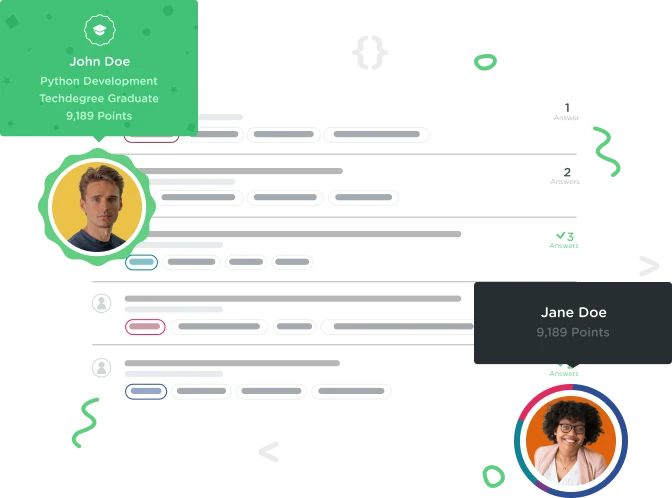
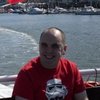
Sean Flanagan
33,235 PointsC# Basics, Final, Task 3
Hi. I'm supposed to add input validation for when a non-positive number is input but it won't pass.
Here's my attempt:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int counter = 0;
String times = Console.ReadLine();
try
{
int totals = int.Parse(times);
while (counter < totals)
{
Console.Write("\"Yay!\"! ");
counter += 1;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
if (totals <= 0) //My attempt at Task 3
{
Console.WriteLine("You must enter a positive number.");
continue;
}
}
}
}
Thanks for any help.
2 Answers

Pablo Rocha
10,142 PointsYou need to move your if statement inside the try statement. Reason is that you are currently attempting to write to the screen before you are checking for a negative number. You need to verify the input is positive and then you can write to the screen.
Another thing, you are using a continue statement and that is only valid inside a loop/while. Use a return statement instead. If you want to prompt the user again after they enter a negative number, then use a continue but you would need to wrap the entire thing in a while statement with a return statement at the end after all the "Yay!"s are written out.
Your code modified (not wrapped in a while statement):
Console.Write("Enter the number of times to print \"Yay!\": ");
int counter = 0;
String times = Console.ReadLine();
try
{
int totals = int.Parse(times);
if (totals <= 0) //My attempt at Task 3 - this is now within the try statement
{
Console.WriteLine("You must enter a positive number.");
return; // this cannot be a continue statement unless you wrap the the whole thing in a while statement
}
while (counter < totals)
{
Console.WriteLine("\"Yay!\""); // I changed this a bit, you had two exclamation points printing out; changed to WriteLine, the output looks cleaner than just using Write
counter++; // this is a shorter version of what you had, either way is correct
}
} catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
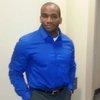
Jonathan Wade Jr
1,051 PointsThanks that helped. i had my if statement after the first initial counter. but after looking at this i see now what i did wrong. This is what worked for me now
class Program //Program will print Yay! x times depending on what number you input { static void Main(string[] args) {
while (true) // if the condition is true the code runs
{
int counter = 0;
int result;
Console.Write("Enter the number of times to print \"Yay!\": ");
try // This one catches Validation error
{
result = Convert.ToInt32(Console.ReadLine());
if (result <= counter)// put at first try method becuase the condition needs to be checked before proceeding
{
Console.WriteLine("You must enter a positive number.");
return;
}
while (result > counter)
{
Console.WriteLine("Yay!");
counter++;
//added the if to tell message about neededing positive number
}
}
catch (FormatException) //catches the exception error
{
Console.WriteLine("You must enter a whole number.");
break;
}
Console.ReadLine();
}
}
}
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Pablo. Thanks for your help. You've earned an up vote and Best Answer.
Sean :-)