Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial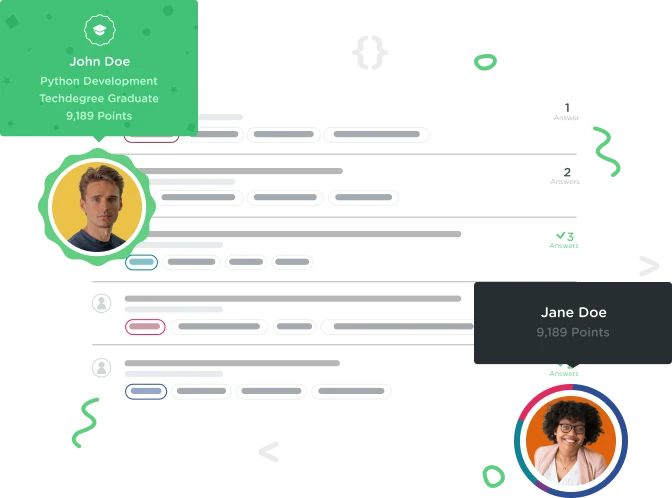
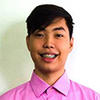
christian lian huat toh
7,213 PointsC# Challenge ( ADDRESS BOOK )
Hi! I've been studying C# and the principles of OOP, I've been challenged to make a program ADDRESS BOOK and maybe I could ask for your help :D, I want to know if the code I've written is a good practice or bad practice, please guide me :'(
THANK YOU VERY MUCH :) BLESS You :D :D
PROBLEM : Address Book
Write a program that functions as an address book. It should have entries containing at least the following information: first and last name, phone number, address, and email address. At a minimum you’ll want an address book entry class. You should be able to add entries and search for entries (by last name at a minimum).
Program.cs
using System;
namespace Treehouse
{
class Program
{
public static void Main()
{
AddressBookEntry AddressBook = new AddressBookEntry(50);
Console.WriteLine("Address book options:\n"+
"add - to add new entry.\n"+
"search - to search entry.\n"+
"quit - to leave.\n"+
"clear - to clear screen\n"+
"options - display instructions\n");
while(true)
{
Console.Write("Option: ");
string Instruction = Console.ReadLine();
if(Instruction.ToLower() == "quit")
{
Console.Clear();
Console.WriteLine("GoodBye!");
break;
}
else if(Instruction.ToLower() == "add")
{
Console.Clear();
AddressBook.NewEntry();
}
else if(Instruction.ToLower() == "search")
{
Console.Clear();
AddressBook.SearchEntry();
}
else if(Instruction.ToLower() == "clear")
{
Console.Clear();
}
else if(Instruction.ToLower() == "options")
{
Console.Clear();
Console.WriteLine("Address book options:\n"+
"add - to add new entry.\n"+
"search - to search entry.\n"+
"quit - to leave.\n"+
"clear - to clear screen\n"+
"options - display instructions\n");
}
else
{
Console.WriteLine("Invalid Input!");
}
}
}
}
}
Person.cs
using System;
namespace Treehouse
{
class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string PhoneNumber { get; set; }
public string HomeAddress { get; set; }
public string EmailAddress { get; set; }
public string GetInfo()
{
string message = "First Name: {0}\nLast Name: {1}\nPhone Number: {2}\nHome Address: {3}\nEmail Address: {4}\n";
return string.Format(message,FirstName,LastName,PhoneNumber,HomeAddress,EmailAddress);
}
}
}
AddressBookEntry.cs
using System;
namespace Treehouse
{
class AddressBookEntry
{
private Person[] _entries;
private int _totalEntries = 0;
public int Size { get; private set; }
public AddressBookEntry(int size)
{
_entries = new Person[size];
Size = size;
}
public void NewEntry()
{
if(_totalEntries < _entries.Length)
{
Console.WriteLine("New Entry:");
Person person = new Person() {
FirstName = InputValidator.LetterInput("First Name: ", "Input should only be letters please try again."),
LastName = InputValidator.LetterInput("Last Name: ", "Input should only be letters please try again."),
PhoneNumber = InputValidator.NumberInput("Phone Number: ", "Input should only be number please try again."),
HomeAddress = InputValidator.AnyInput("Home Address: "),
EmailAddress = InputValidator.AnyInput("Email Address: ")
};
_entries[_totalEntries++] = person;
Console.WriteLine("Added successfuly!");
}
else
{
Console.WriteLine("Address Book is already full.");
}
}
public void SearchEntry()
{
Console.Write("Type the number of the field you want to search\n"+
"[1] First Name \n[2] Last Name \n");
string result = "No result found!";
string Option;
while(true)
{
Option = InputValidator.NumberInput("Option: ", "Input should only be number please try again.");
if(Option == "1" || Option == "2")
{
break;
}
Console.WriteLine("Invalid Input.");
}
if(_totalEntries == 0)
{
result = "Address Book is Empty.";
}
else
{
for(int i = 0; i < _totalEntries; i++)
{
Person person = _entries[i];
if(Option == "1")
{
string search = InputValidator.LetterInput("First Name: ", "Input should only be letters please try again.");
if(person.FirstName.ToLower() == search.ToLower())
{
result = person.GetInfo();
break;
}
}
else if(Option == "2")
{
string search = InputValidator.LetterInput("Last Name: ", "Input should only be letters please try again.");
if(person.LastName.ToLower() == search.ToLower())
{
result = person.GetInfo();
break;
}
}
}
}
Console.WriteLine(result);
}
}
}
InputValidator.cs
using System;
using System.Text.RegularExpressions;
namespace Treehouse
{
static class InputValidator
{
public static string AnyInput(string label = "Input string: ",
string errorMessage = "Input must only be letters.")
{
string input;
Console.Write(label);
input = Console.ReadLine();
return input;
}
public static string LetterInput(string label = "Input string: ",
string errorMessage = "Input must only be letters.")
{
string input;
while(true)
{
Console.Write(label);
input = Console.ReadLine();
if(!(new Regex("[0-9]").IsMatch(input)))
{
break;
}
Console.WriteLine(errorMessage);
}
return input;
}
public static string NumberInput(string label = "Input string: ",
string errorMessage = "Input must only be numbers.")
{
string input;
while(true)
{
Console.Write(label);
input = Console.ReadLine();
if(!(new Regex("[a-zA-Z]").IsMatch(input)))
{
break;
}
Console.WriteLine(errorMessage);
}
return input;
}
}
}
1 Answer

Jon Wood
9,884 PointsThis looks great! I only have a few nitpicks, really, that I can tell.
- The Person.GetInfo looks like it can be refactored to just override the
ToString
method. - For the regex in
InputValidator
, I wonder if you could just use aint.TryParse
instead. There's a famous quote about regex from Jamie Zawinski that always comes to mind when I think about it:
Some people, when confronted with a problem, think "I know, I'll use regular expressions." Now they have two problems.
I can't wait for you to get into LINQ and other data structures like lists. Those may make you want to refactor this :p.
christian lian huat toh
7,213 Pointschristian lian huat toh
7,213 PointsThank you so much sir, I've learned something new again :), I'm looking forward in learning other awesome features c# offers and I'm planning to take the ASP.NET web development after the c# course :), hopefully I'll be able to find a job with the knowledge that I have right now.