Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial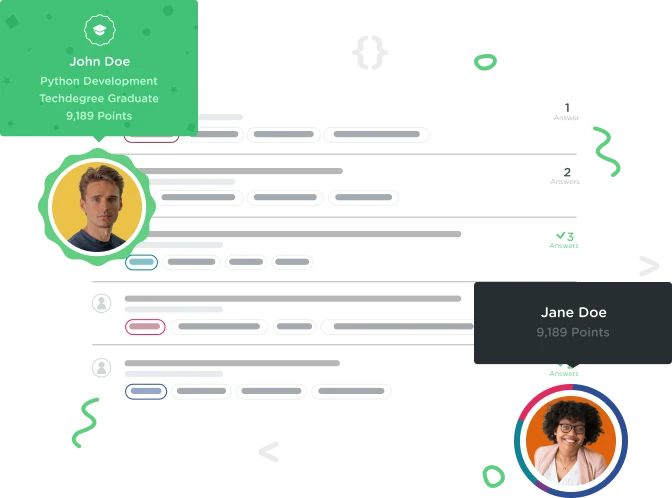

Eric Scott
1,754 PointsC# Code Challenge 2 prints out infinite not input number of times
Why does my code print out infinite Yay! instead of user input number of times I press Enter and Yay! is printed out the number of times I press Enter.
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": "); int numberCount = 0; try { numberCount = Convert.ToInt32(Console.ReadLine()); } catch (FormatException fe) { Console.Write("You must enter a whole number."); Console.ReadLine(); } while (numberCount > 0) { numberCount =+1; Console.Write("Yay!"); Console.ReadLine(); } } }
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int numberCount = 0;
try
{
numberCount = Convert.ToInt32(Console.ReadLine());
}
catch (FormatException fe)
{
Console.Write("You must enter a whole number.");
Console.ReadLine();
}
while (numberCount > 0)
{
numberCount =+1;
Console.Write("Yay!");
Console.ReadLine();
}
}
}
}
2 Answers
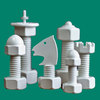
Steven Parker
241,970 Points
You're comparing the count against 0.
So no matter how many times you increment it in the loop, it will still be greater than 0 (until you exceed the maximum numeric storage value).
You might want to use a separate value to hold the user's limit, and and then compare the limit to the count:
// while (numberCount > 0) <- so instead of this...
while (numberCount < user_specified_limit) // ...do something like this
I assume you added the ReadLine after they "Yay!" just for testing. Be sure to remove it for the challenge.
Also, be aware that if an error occurs, the challenge expects the program to print the error message out and then end, so so you don't want another ReadLine after the error. And be sure you don't print out any "Yay!"s in that case, either.

Eric Scott
1,754 PointsThanks Steven I was attempting different combinations of the user and increment values. I will try this out and see how it works. Also tried to use =+ 1. I felt I was close but just missing something simple that I am unaware of. Appreciate your time and effort.
Eric Scott
1,754 PointsEric Scott
1,754 PointsThanks for your reply I am not sure what you meant when you wrote "You might want to use a separate value to hold the user's limit, and and then compare the limit to the count:" So what I did was use the while loop but the code would skip over the and end after the userSpecfied = numberCount and end. No print out of Yay. Then I removed the while and the code would print out Yah but only one time. This is what I experienced before the multi print ability of the string Yay from my original post.
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": "); int numberCount = 0; int userSpecifiedValue; try { userSpecifiedValue = Convert.ToInt32(Console.ReadLine()); } catch (FormatException fe) { Console.Write("You must enter a whole number."); Console.ReadLine(); } userSpecifiedValue = numberCount; userSpecifiedValue ++; Console.Write("Yay!"); Console.ReadLine(); } } }
Steven Parker
241,970 PointsSteven Parker
241,970 PointsYou definitely need the loop to print the right number of times!
You must have had that working before to pass task 1, right? But you're pretty close if you put the loop back, and increment the count instead of the limit:
Check the Markdown Cheatsheet link below for info on formatting code.