Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial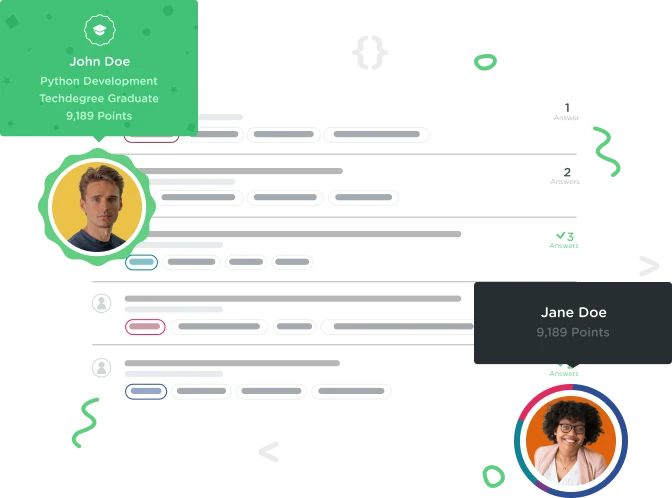

Larry Singleton
18,947 PointsC# Collections
Lost, please point me in the right direction, thanks.
Question: Create a static method named GetPowersOf2 that returns a list of integers of type List<int> containing the powers of 2 from 0 to the value passed in. For example, if 4 is passed in, the method should return this list of integers: { 1, 2, 4, 8, 16 }. The System.Math.Pow method will come in handy.
Link: https://teamtreehouse.com/library/c-collections/lists/lists-2
One of my many attempted answers: namespace Treehouse.CodeChallenges { public static class MathHelpers { public static List<int> GetPowersOf2(int input) { var returnList = new List<int>(); for (int i = 0; i < input + 1; i++) { returnList.Add((int)System.Math.Pow(2, i)); } return returnList;
}
}
}
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<int> GetPowersOf2(int input)
{
var returnList = new List<int>();
for (int i = 0; i < input + 1; i++)
{
returnList.Add((int)System.Math.Pow(2, i));
}
return returnList;
}
}
}
3 Answers
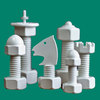
Steven Parker
241,970 PointsCheck the "preview" button when you have compile issues.
In this case, you would have seen a message reminding you that you had forgotten to include a line like this in your file:
using System.Collections.Generic;
That's the namespace that "List" is defined in.
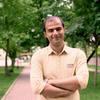
S.Amir Nahravan
4,008 PointsYou can use this code
using System.Collections.Generic; namespace Treehouse.CodeChallenges { public static class MathHelpers { public static List<int> GetPowersOf2(int powers) { List<int> result = new List<int>(); int po; for(int i=0; i<=powers; i++) { po = (int)System.Math.Pow(2,i); result.Add(po); } return result; } } }

Larry Singleton
18,947 PointsYeah, I found that. I couldn't figure out how to get back to this help question to place a "Never mind, I figured it out" message. Thanks for the help though.