Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial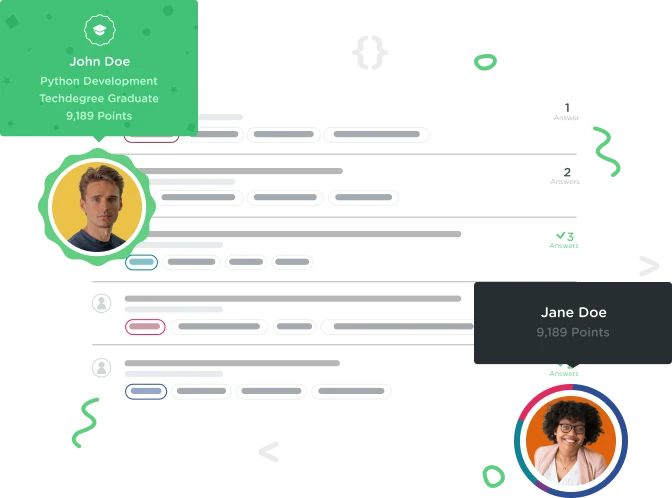
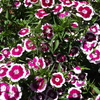
Allison Cuyjet
20,689 PointsC# Collections Challenge Task (Jagged Arrays)
I seem to be stuck on this challenge. When I preview my code, I don't get any errors, but when I submit my work, I get a Bummer! message telling me that an "object reference not set to an instance of an object." So I know I've got an unhandled NullReferenceException, but I'm not clear on how to resolve it.
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
int[][] multiplicationTable = new int[maxFactor][];
for (int row = 0; row <= maxFactor; row++)
{
for (int column = 0; column <= maxFactor; column++)
{
multiplicationTable[row][column] = row * column;
}
}
return multiplicationTable;
}
}
}
3 Answers

Lukas Dahlberg
53,736 PointsAh, right.
So, I missed a couple things above. Let's try again.
First, you didn't initialize the column rows above. And when you do the calculations, you'll find that maxFactor is one short of what you'll require because of the 0s.
So the solution looks like this:
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
maxFactor = maxFactor + 1;
int[][] multiplicationTable = new int[maxFactor][];
for (int row = 0; row < multiplicationTable.Length; row++)
{
multiplicationTable[row] = new int[maxFactor];
for (int column = 0; column < multiplicationTable[row].Length; column++)
{
multiplicationTable[row][column] = row * column;
}
}
return multiplicationTable;
}
}
}
Personally, I find it easiest to do the solutions in Visual Studio 2015 and port them over.
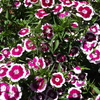
Allison Cuyjet
20,689 PointsThank you! That makes sense.

Lukas Dahlberg
53,736 PointsYou don't want to use maxFactor to set your for loop limit.
So instead of:
for (int row = 0; row <= maxFactor; row++)
for (int column = 0; column <= maxFactor; column++)
use:
for (int row = 0; row < multiplicationTable.Length; row++)
for (int column = 0; column < multiplicationTable[row].Length; column++)
(in their respective spots, of course.)