Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial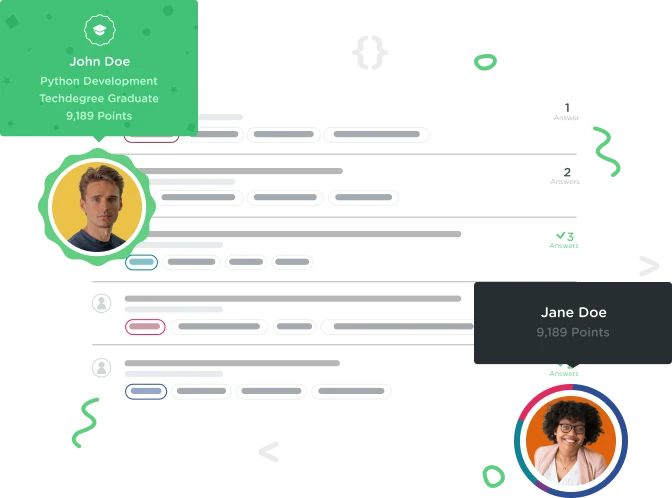

Mitchell Sawicki
1,240 PointsC# Final part 3 of 3. How does my code look? All I added since part 2 was an "if/else" statement. Thanks much!
Since i have only added an "if/else" statement to the code I think it should work but a second set of eyes would be of benefit. Those more experienced than mine in particular!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var numOfTimes = Console.ReadLine();
try
{
int num = int.Parse(numOfTimes);
if (numOfTimes < 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
for (int i = 0; int < num; int ++)
{
Console.WriteLine("\"Yay!\"");
}
}
}
catch (System.FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
1 Answer
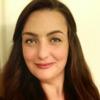
Jennifer Nordell
Treehouse TeacherHi there! I think you're doing fairly well, but the biggest problem here is your for loop. I believe it must have been edited somehow as I don't believe it would have or should have passed the first step. The int
you are using is a keyword reserved by C# for the integer data type. But you should be comparing an integer here (namely num
). I edited your code so that it passes, and will go through it with you a bit.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var numOfTimes = Console.ReadLine(); // here you read in a number from the user as a string
try
{
int num = int.Parse(numOfTimes); // we're trying to parse a number out of the string they entered
if (num < 0) // If the number was negative
{
Console.WriteLine("You must enter a positive number."); // tell them they need a positive number
}
else // otherwise
{
for (int i = 0; i < num; i++) // start a counter at 0. While the counter is less than the number we parsed out, increment the counter
{
Console.WriteLine("\"Yay!\""); //Write out Yay!
}
}
}
catch (System.FormatException)
{
Console.WriteLine("You must enter a whole number."); //If the number could not be parsed, tell the user they must enter a whole number
}
}
}
}
Hope this helps!
Mitchell Sawicki
1,240 PointsMitchell Sawicki
1,240 PointsThis was very helpful, thanks much! The explanations that were next to each line of code helped me understand the process better.