Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial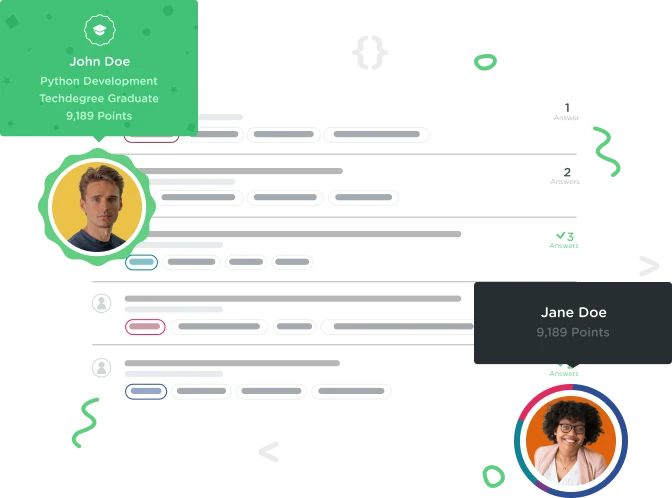

Mark Libario
9,003 PointsC# Interactive quiz challenge, how do i create a grade percentage aside from just showing the # of correct answers.
using System;
namespace Interactive.Quiz
{
class Program
{
static void Main()
{
var correctAnswers = 0;
var totalQuestions = 5;
Console.WriteLine ("Welcome to Mark's Interactive Quiz!");
Console.WriteLine ("To begin with, please input your name.");
var userName = Console.ReadLine ();
Console.WriteLine ("Hello, " + userName + ". May the odds be in your favor.");
Console.WriteLine (" Question #1 : ");
var userAnswer1 = Console.ReadLine ();
Console.WriteLine (" Question #2 : ");
var userAnswer2 = Console.ReadLine ();
Console.WriteLine (" Question #3 : ");
var userAnswer3 = Console.ReadLine ();
Console.WriteLine (" Question #4 : ");
var userAnswer4 = Console.ReadLine ();
Console.WriteLine (" Question #5 : ");
var userAnswer5 = Console.ReadLine ();
if (userAnswer1.ToLower () == "one")
{
correctAnswers ++;
}
if (userAnswer2.ToLower () == "two")
{
correctAnswers ++;
}
if (userAnswer3.ToLower () == "3" ||userAnswer3.ToLower () == "three" )
{
correctAnswers ++;
}
if (userAnswer4.ToLower () == "four")
{
correctAnswers ++;
}
if (userAnswer5.ToLower () == "five")
{
correctAnswers ++;
}
Console.WriteLine ("Your total correct answers: " + correctAnswers + ".");
Console.WriteLine ("You got a grade of " + (correctAnswers/totalQuestions*100));
}
}
}
the last line I wrote, doesnt convert anything to percentage. It just shows 0.
1 Answer
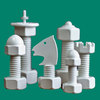
Steven Parker
231,124 PointsWell, it doesn't always show 0.
If you get everything right, it shows 100. This is because of the integer math operations. Anything divided by something larger than itself will yield 0 in integer math. Then multiplying 0 by 100 is still 0.
So just perform the operations in the other order:
Console.WriteLine ("You got a grade of " + (correctAnswers*100/totalQuestions));