Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial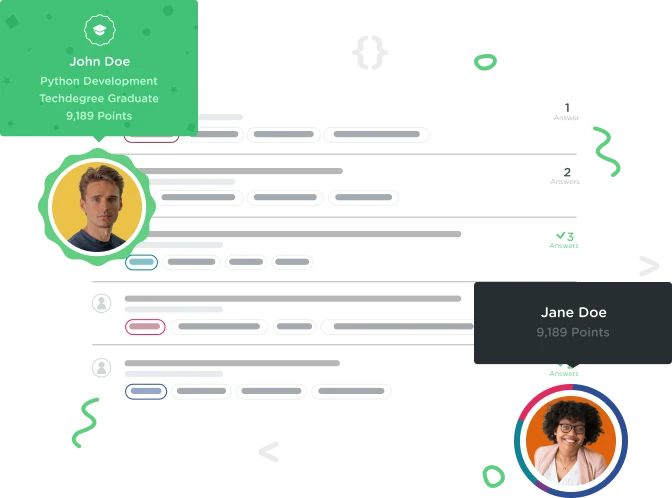
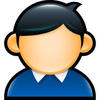
Daniel Hildreth
16,170 PointsC# Multidimensional Arrays Challenge
I need help understanding the multidimensional array, so I can pass this challenge. I've looked at code examples on MSDN, but none of them have it where you pass it your own values like the maxFactor used in the challenge. They all have it where you instantiate them with numbers.
Below is my code that I need help with.
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
maxFactor = maxFactor + 1;
int[,] table = new table[maxFactor][];
for(var row = 0; row <= table.Length; row++)
{
for(var col = 0; col <= table.Length; col++)
{
table[row][col] = row * col;
}
}
return table;
}
}
}
9 Answers
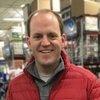
Josh Franey
2,159 PointsDaniel,
In the example output that the challenge shows, when maxFactor = 3, you end up with a 2-dimensional array that is 4 rows and 4 columns. So, your line: int[,] multiplicationTable = new int[maxFactor, maxFactorPlusOne];
would have a different number of rows and columns. Hint: You are on the right track with maxFactorPlusOne.
The length property of a multidimensional array gives you the total length. So, if maxFactor = 3, our 4x4 array would have a length of 16. That's not what you want to use in your nested for loops. But, multidimensional arrays have a nice helper method to get the length of a specific dimension called GetLength(n). So, multiplicationTable.GetLength(0) should give you the length of the first dimension (number of rows), and multiplicationTable.GetLength(1) should give you the length of the second (number of columns). We also know that the result in our case will always be 4 (when maxFactor is 3). So, you could technically use a different value in your loops instead of looking at the size of your array. Maybe one that you already calculated? ;)

Azim Sodikov
11,432 Pointsnamespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
int[,] arr = new int[maxFactor + 1, maxFactor +1];
for (int x = 0; x <= maxFactor; x++){
for (int y = 0; y <= maxFactor; y++)
{
arr[x, y] = x * y;
}
}
return arr;
}
}
}
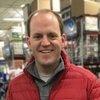
Josh Franey
2,159 PointsThomas Beaudry and Amy Shah ... both of you mentioned that you were having issues with this one over the weekend. My original answer to Daniel Hildreth was a bit vague because he asked "Can you explain to me what I'm doing wrong, and what I need to do to fix it without giving the answer away?" So, my answer was specific to helping him get over the hump based on the code he'd already written. Here's a more generic explanation. Hope it helps.
There are several ways to write this. You could use the fact that you always know the length and width up front to avoid ever having to look at the array itself to see how big it is. Also, since length and width are the same (i.e. it's not a jagged array), you can use the same variable for both. So your loops like like this...
for (int row = 0; row < maxFactorPlusOne; row++)
for (int column = 0; column < maxFactorPlusOne; column++)
You can also look back at the array that you just declared (and instantiated with a fixed size). You must use the Array.GetLength() method, passing the index of the dimension (i.e. 0 for rows, 1 for columns in our case). Array.Length is no help because it will return the total size of the array. (i.e. For a 4x4 2-dimensional array, Array.Length will give you 16.) Using Array.GetLength() is more generic than the code above, but gives up a tiny bit of performance to solve a problem that you already know the answer to. After all, you are the one who just created the array! You already know how big it is! However, if you didn't already have knowledge about the size of the array, this would be better...
for (int row = 0; row < multiplicationTable.GetLength(0); row++)
for (int column = 0; column < multiplicationTable.GetLength(1); column++)
Here it is when you put it all together the first way (in this case, the better way)...
public static int[,] BuildMultiplicationTable(int maxFactor)
{
//Since we know that we need to include zero, assuming maxFactor = 3, our rows and
//columns will go from 0 to 3. So, they will both have a length of 4 (0 1 2 3).
//So, we always need to add one to maxFactor to account for zero.
var maxFactorPlusOne = maxFactor + 1;
//Declare a (2-dimensional) multidimensional array with the right amount of rows and columns.
int[,] multiplicationTable = new int[maxFactorPlusOne, maxFactorPlusOne];
//The outer loop will iterate the rows (0 1 2 3).
for (int row = 0; row < maxFactorPlusOne; row++)
{
//The inner loop will iterate the columns (0 1 2 3).
for (int column = 0; column < maxFactorPlusOne; column++)
{
multiplicationTable[row, column] = row * column;
}
}
return multiplicationTable;
}
And, here is some code to put into your Console app to make it print out pretty like they showed in the instructions. Note: My code that writes the output assumes that it has no knowledge of the size of the array...
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Press <Enter> to begin.");
Console.ReadLine();
int maxFactor = 3;
var multiplicationTable = MathHelpers.BuildMultiplicationTable(maxFactor);
for (int row = 0; row < multiplicationTable.GetLength(0); row++)
{
for (int column = 0; column < multiplicationTable.GetLength(1); column++)
{
Console.Write(multiplicationTable[row, column] + " ");
}
Console.WriteLine();
}
Console.WriteLine("Press <Enter> to exit.");
Console.ReadLine();
}
}
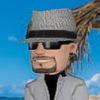
Thomas Beaudry
29,084 PointsGood "Looking out" Josh, I think you more than cleared up this challenge, What I did was I went on the MDN and figured it out from some of their examples , great answer and thank you!
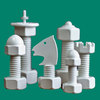
Steven Parker
241,970 Points
Your array is still a bit too small.
When you sized your array:
int[,] multiplicationTable = new int[maxFactor, maxFactorPlusOne];
You forgot that the array should be square, which means that both dimensions should be maxFactorPlusOne.
Also, you will probably want to use that same value in both of your loop comparisons. Right now, your first loop is comparing to the array length, which is much more than a single row. And your second loop is using the value from one of the cells (which has not been initialized yet).
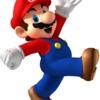
Terry McDuffie
12,786 PointsDaniel Hildreth did you ever solve this?
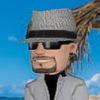
Thomas Beaudry
29,084 PointsI've gone over this challenge quite a few times and still don't get it??
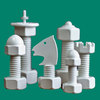
Steven Parker
241,970 Points
You have mixed multidimensional syntax with jagged array syntax.
Jagged arrays have multiple sets of brackets (one for each dimension), but multidimensional arrays have only one set of brackets, with the dimensional terms separated by commas.
Also, while it works, it's not a good practice to modify passed-in arguments. So instead of this:
maxFactor = maxFactor + 1;
you might have something like this:
var maxFactorPlusOne = maxFactor + 1;
Then, you would refer to maxFactorPlusOne in all the following statements.
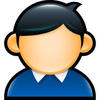
Daniel Hildreth
16,170 PointsHey Steven,
I'm still a little confused as about the syntax of the multidimensional arrays. I have this code, but it says the index was out of bounds. Can you explain to me what I'm doing wrong, and what I need to do to fix it without giving the answer away? BTW, here is the link to the challenge in case you need to look at it:
https://teamtreehouse.com/library/c-collections/arrays/multidimensional-arrays
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
var maxFactorPlusOne = maxFactor + 1;
int[,] multiplicationTable = new int[maxFactor, maxFactorPlusOne];
for (int row = 0; row < multiplicationTable.Length; row++)
{
for (int column = 0; column < multiplicationTable[row, maxFactorPlusOne]; column++)
{
multiplicationTable[row, column] = row * column;
}
}
return multiplicationTable;
}
}
}
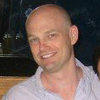
Noah Yasskin
23,947 PointsHere's my solution:
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
int[,] table = new int[maxFactor + 1, maxFactor +1];
for(int x = 0; x <= maxFactor; x++)
{
for(int y = 0; y <= maxFactor; y++)
{
table[x, y] = x * y;
}
}
return table;
}
}
}

Greg Heffley
6,371 PointsHello,
I'm learning C# and am wondering why do we need to use maxFactor + 1?

Nicholas Izosimov
5,728 PointsBecause the computer calculates from 0. So 2 in an array is actually = 1. +1 calibrates this particular method. This is my understanding at least.