Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial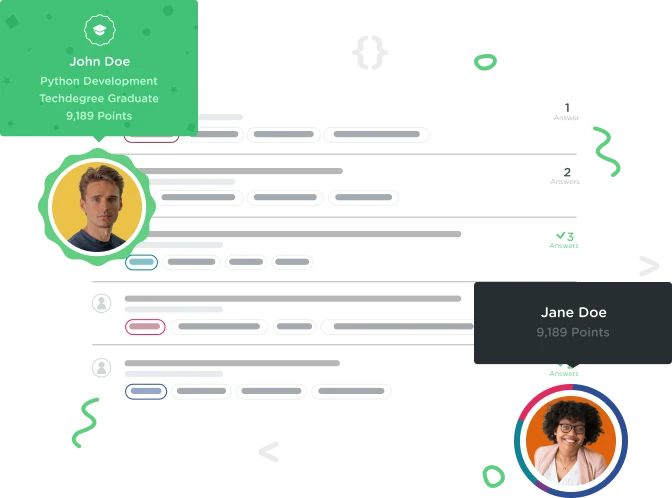
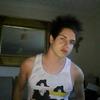
Matthew Smart
12,567 PointsC# Objects - creating address book from teachers notes
Hello, all!
So I've just finished the C# objects course with the TreehouseDefense game. Great course, and feel like I learned well. In the teacher's notes, Jeremy McLain left a few ideas to play around with to get used to c# more.
So I'm having a go at the Address Book. So far I have built in the functionality to add someone to the address book, however, I feel like I am probably going the wrong way about it. (I've picked up some bad habits in PHP)
Can you guys take a look at what I have done so far and see if I'm going in the right direction, or if I should be doing things differently?
AddressBook.cs :
namespace AddressBook
{
class AddressBook
{
public string FirstName { get; private set; }
public string LastName { get; private set; }
public long PhoneNumber { get; private set; }
public string Address { get; private set; }
public string EmailAddress { get; private set; }
public AddressBook(string firstName,string lastName,long phoneNumber,string address,string emailAddress)
{
FirstName = firstName;
LastName = lastName;
PhoneNumber = phoneNumber;
Address = address;
EmailAddress = emailAddress;
}
}
}
Person.cs :
namespace AddressBook
{
class Person : AddressBook
{
public Person(string firstName, string lastName, long phoneNumber, string address, string emailAddress) : base(firstName, lastName, phoneNumber, address, emailAddress)
{
}
}
}
Program.cs :
using System;
namespace AddressBook
{
class Program
{
static void Main()
{
Program program = new Program();
program.createAddressBook();
Console.WriteLine("Welcome to your Address Book");
program.addOrSearch();
}
public void createAddressBook()
{
Person[] persons =
{
new Person("Name", "Last Name", 077077707707, "address line", "email@gmail.com"),
new Person("Rhian", "Johnson", 077077707707, "address line", "email@hotmail.com")
};
}
public void addOrSearch()
{
Console.WriteLine("Would you like to search or add a person in your Address Book? (search/add)");
string response = Console.ReadLine();
if(response.ToLower() == "search")
{
this.searchAddressBook();
}
else if(response.ToLower() == "add")
{
this.addToAddressBook();
}
else
{
Console.WriteLine("Your must enter either 'search' or 'add' to continue");
this.addOrSearch();
}
}
public void addToAddressBook()
{
Console.WriteLine("Enter the first name");
string firstName = Console.ReadLine();
Console.WriteLine("Enter the last name");
string lastName = Console.ReadLine();
Console.WriteLine("Enter the phone number");
string phoneNumber = Console.ReadLine();
Console.WriteLine("Enter the address");
string address = Console.ReadLine();
Console.WriteLine("Enter the email address");
string emailAddress = Console.ReadLine();
Person person = new Person(firstName, lastName, Convert.ToInt64(phoneNumber), address, emailAddress);
Console.WriteLine("");
Console.WriteLine("We have added - " + person.FirstName + " " + person.LastName + " to the database");
Console.WriteLine("");
this.addOrSearch();
}
}
}
1 Answer

James Bergen
9,037 PointsI don't think that Person should inherit from AddressBook. I think that you should rename Person to PersonEntry. AddressBook would then have a property that is a collection of PersonEntry objects (ex: List<PersonEntry>). In your addToAddressBook method, you can add the entry to the AddressBook PersonEntry collection. You could then serialize the AddressBook object to save the state to a file.
With this approach in your search method, you can easily use LINQ to search the PersonEntry collection.
I think you should make PhoneNumber a string.
I don't see an option to quit. You should add that as well.
Steven Parker
231,122 PointsSteven Parker
231,122 PointsIt would be easier for someone to examine your project if you make a snapshot of your workspace and post the link to it here.