Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial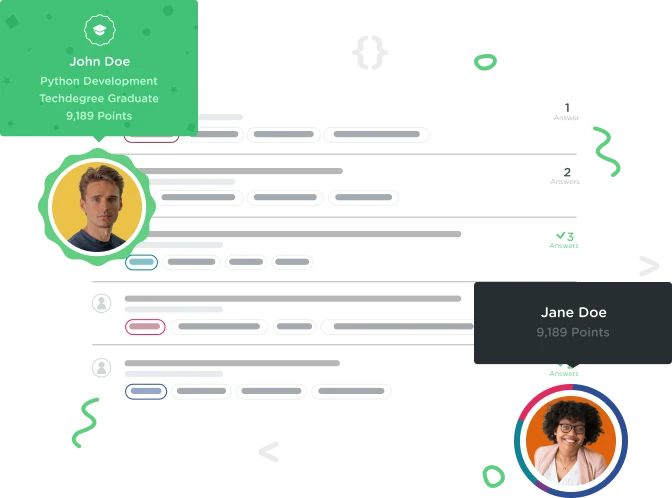

Stephan Olsen
6,650 PointsC# Validating user input to numbers and positive numbers
I've almost completed the C# Basics tutorial. I'm however stuck on task 3, where I have to validate the user input to make my code accept positive numbers only, otherwise it should return a string to the user.
To troubleshoot more easily I copied my code from task 1 and 2 into Visual Studio, so I could preview the console application there. After making changes the code seems to be doing what it should...
Any help is appreciated!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
while (true)
{
try
{
var entry = Console.ReadLine();
var amountsOfYay = int.Parse(entry);
if (amountsOfYay <= 0)
{
Console.Write("You must enter a positive number.");
continue;
}
var counter = 0;
while (amountsOfYay > counter)
{
Console.Write("Yay! \n");
counter += 1;
}
}
catch (FormatException)
{
Console.Write("You must enter a whole number.");
continue;
}
break;
}
}
}
}
1 Answer

andren
28,558 PointsThere is nothing technically wrong with your code, you are very thorough and the program is well designed, if this was a challenge issued by and evaluated by a human it would likely pass.
But it's not evaluated by a human but by a machine, and that machine expects the code to do exactly what it instructed you to do and nothing more, the task asks that you check to see if a number is negative and print a message if it is, but that is also all that it asks.
It does not ask you to add a loop that continually prompts for new input when the number given is wrong, logically adding such a loop is correct, and if you were making this program for real, most people would indeed add one. But that doesn't change the fact that the challenge never asks you to do it, and therefore it doesn't expect you to do it either.
The solution to this challenge is therefore far simpler than the code you actually wrote, all you needed to to do pass task 3 is to literally just check to see if the number is negative and print the "You must enter a positive number." message which you did, but you also added a loop along with continue and break statements, this crashes your code due to the fact that the treehouse challenge only provides the program with input once, so asking for input multiple times causes problems.
Here is the solution code:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
var entry = Console.ReadLine();
var amountsOfYay = int.Parse(entry);
if (amountsOfYay < 0)
{
Console.Write("You must enter a positive number.");
}
var counter = 0;
while (amountsOfYay > counter)
{
Console.Write("Yay! \n");
counter += 1;
}
}
catch (FormatException)
{
Console.Write("You must enter a whole number.");
}
}
}
}
Stephan Olsen
6,650 PointsStephan Olsen
6,650 PointsYou're absolutely right!