Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial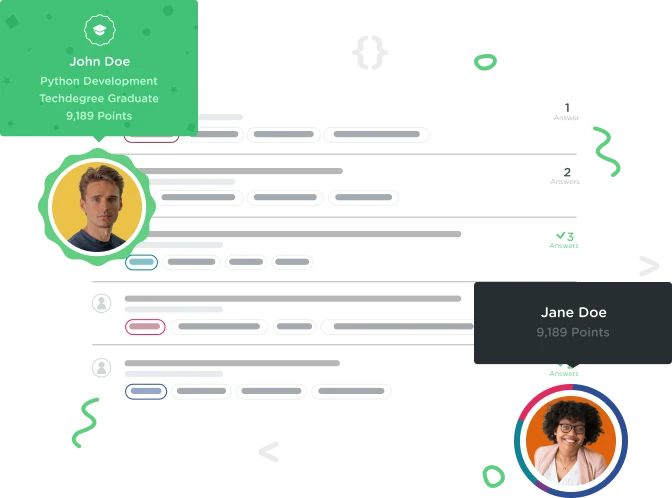
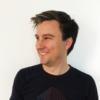
Christian Lawrence
3,941 PointsC# While yay <= 5
I can't seem to get the console to write out "Yay" five times. It's as if the while is only printing out once
' Bummer! I entered 5, but saw Yay! printed 1 time.'
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
int numTimes = Int32.Parse(entry);
while(numTimes <= 5)
{
Console.Write("Yay " + numTimes);
numTimes++;
}
}
}
}
2 Answers

Gavin Ralston
28,770 Pointsint numTimes = Int32.Parse(entry);
while(numTimes <= 5)
{
Console.Write("Yay " + numTimes);
numTimes++;
}
So you parse the entry "5" and store it in numTimes...
Then you say "while numTimes is less than or equal to 5" which is is (once! it's already 5), then print "Yay" and the number of times. If you entered 6 or higher, it wouldn't print anything at all.
So you enter 5, it's less than or equal to 5, you print, then increment it to 6, which will end the loop.
Try reducing numTimes in your loop, count down to 0, for instance.
while(numTimes > 0) {
// do your thing here
numtimes--;
}
Or set a sentinel value like "timesPrinted" to 0, and then
while(timesPrinted < numTimes) {
//do your thing
//increment the number of times printed
}
Then it'll run the loop til the number of times you printed the statement matches the number of times they want to say "Yay" :)

Albert Tomasura
11,041 PointsI love how Python can produce the same results with two lines of code. It's no problem to multiply a string by an int, no conversions necessary

Gavin Ralston
28,770 PointsPython's fun like that. :)
That being said...
def cheer(num):
print("Yay!\n" * num)
Has a bit of trouble with the following:
cheer(-1)
cheer("banana")
Never mind that it would also require someone to have Python installed in order to import and run it in the interactive shell, or you'd need to add a few lines after if name == "main", and then more lines to unpack args
The C# examples above are, of course, almost as naive, and don't do much more about invalid input, but I think it's safe to say that no matter how dead simple the syntax seems at first, you'll find almost all languages wind up requiring you to expend a few more lines than you thought. :)
Christian Lawrence
3,941 PointsChristian Lawrence
3,941 PointsCheers Gavin,
I got it to work, think I was getting mixed with a for loop.
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsIt happens.
Like, for me, all the time. :)