Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial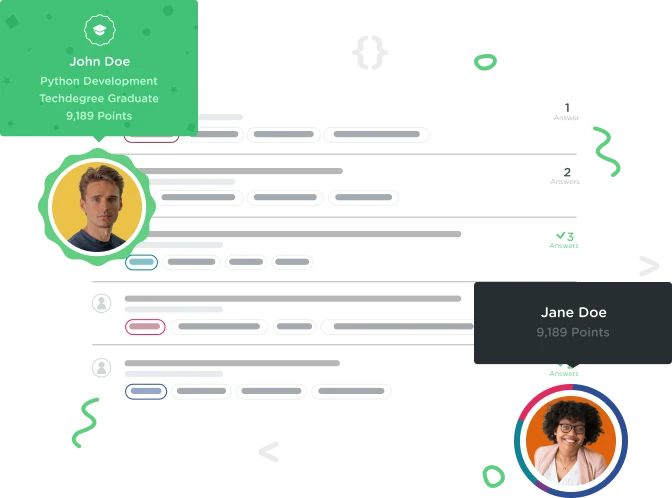
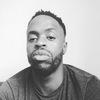
olu adesina
23,007 Pointsc# why is my counter & input values variables not holding there values
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace practice_project
{
class Averager
{
static void Main(string[] args)
{
while (true)
{
int counter = 0;
Console.Write("Enter a random number: ");
string input = Console.ReadLine();
if (input.ToLower() =="quit")
{
Console.WriteLine(counter);
Console.ReadLine();
break;
}
try
{
int total = int.Parse(input);
counter += 1;
total += total;
}
catch (FormatException)
{
Console.WriteLine("invalid input numbers only");
}
}
}
}
}
2 Answers

andren
28,558 PointsSince you declare the counter
variable and assign it to 0 at the start of the loop, it will have its value reset each time the loop runs.
If you want the count
to increase you have to move its declaration outside the loop.
As far as my testing showed your input
variable does hold it's value just fine. Though I would like to point out that you should also move the declaration of the total
variable outside the loop. Due to variable scope if you declare it within the try
block then it will only be exist within that block of code.
Here is a revised version of your code where I have fixed the two issues I described above:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace practice_project
{
class Averager
{
static void Main(string[] args)
{
int counter = 0; // Declare counter outside loop
int total = 0; // Declare total outside loop
while (true)
{
Console.Write("Enter a random number: ");
string input = Console.ReadLine();
if (input.ToLower() =="quit")
{
Console.WriteLine(counter);
Console.ReadLine();
break;
}
try
{
counter += 1;
total += int.Parse(input); // Add the parsed input to the total variable directly
}
catch (FormatException)
{
Console.WriteLine("invalid input numbers only");
}
}
}
}
}
I also changed the way the input is added to total while I moved it. And added comments describing my changes. With those changes your program should work as you intended, and you can now reference both counter
and total
within the if
statement that runs when you quit the program.
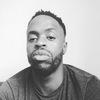
olu adesina
23,007 Pointsthank you it worked well