Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial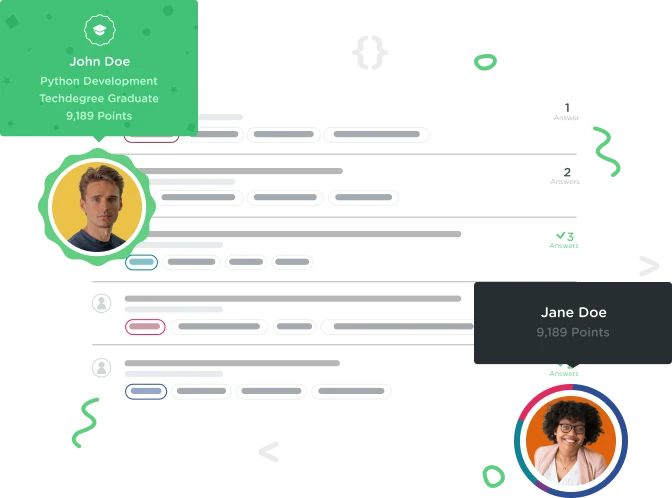

Brahim Farhani
1,534 PointsCan ANYONE give me a GOOD English explanation not too complicated on of initializers ! Thanks :D
Can ANYONE give me a GOOD English explanation not too complicated on of initializers ! Thanks :D
3 Answers

tytyty
4,974 PointsI can give a simple definition because I am new to programming and I teach English in Japan HaHa.
Swift enforces you to initialize every property before it is ever/might ever be used. It’s a safety feature in Swift related to something else in programming called superInit but don’t worry about that.
An initializer is a block of code that is created immediately after objects such as structs, enums or classes.
The main purpose of an initializer is to initialize the properties that have not been initialize yet.
All the properties/attributes have to be initialized or else you will get an error. Again, you cannot construct an object without all of its properties initialized.
Initializers are like special functions/methods that can be used to create a new instance of a particular type. Swift initializers do not return a value. Their primary role is to ensure that new instances of a type are correctly initialized before they are used for the first time. In the example code below “Vehicle” is a type.
An initializer method extends the functionality of objects. If you didn’t want to select a specific member value when creating an instance then you can provide an init method which defaults to one of the member values.
You don’t have to initialize a property in the init method. You can also initialize a property right after the property as shown in the code example below.
Optionals like “String?” are initialized the moment they are declared therefore you don’t need to initialize them inside the initializer.
In the code example below ’name’ is a constant “let name:String”, but actually it can be changed as shown in the code example. This programming feature can only be done in an initializer.
Class Vehicle {
var currentSpeed:Int // you could initialize like this “var currentSpeed:Int = 2”
let name:String
var description:String
var color:String? // Optionals don’t need to be initialized
init() {
currentSpeed = 1 // Good to put in a default value in this game
name = “unknown” // Constants can only be modified here
description = “\(name) running at speed of \(currentSpeed)”
}
}
This Apple documentation is simple and excellent. https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Initialization.html
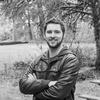
Ryan Ackermann
14,665 PointsHey Brahim,
I would say that an initializer is you, the programming, telling the computer that information is going to be stored and that information is going to be stored in a certain location. For example, if I were to explain to someone the Pythagorean theorem I would first have to explain the basic concepts of mathematics and algebra, thereby initializing the concept of math so they can learn the Pythagorean theorem. So in the shortest way, initializing is assigning a place for information to be stored.
I hope that helps :)
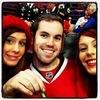
Patrick Cooney
12,216 PointsI would tweak this analogy a little bit. It would be like opening your notebook to a blank page and writing "pythagorean theorem" across the top where you're going to put the notes on the Pythagorean theorem. You're creating a space in your notebook and saying "okay, this page is reserved for notes on the Pythagorean theorem", you're reserving a space so that when you're ready to take the notes you have somewhere to store them. Many times you're also assigning a value to it at the same time.
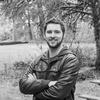
Ryan Ackermann
14,665 PointsI agree Patrick :)
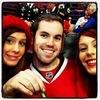
Patrick Cooney
12,216 PointsTo put it in the most basic terms, initializers give the object a starting value. If you're familiar with Java it's like a constructor. Basically it sets up the object so it's not just a null. There is far more complexity to it but that's the very high level idea behind it.

Brahim Farhani
1,534 PointsSwift is the first language am learning, with these videos i kinda find it difficult as the use of difficult terms :(