Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial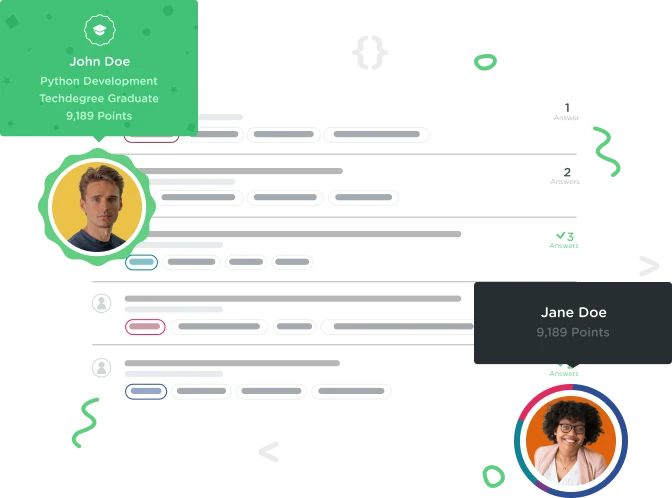

robinhickson
Front End Web Development Techdegree Graduate 18,162 PointsCan I create a collection of class instances using method inside Class template?
I'm attempting to track all instances of a Class by adding a method to the Class template, which would (should) push each instance to an array on creation... (I know I need to access ALL instances later).
const projectsCollection = [];
let Project = class {
constructor(projectname, genre,){
this.projectname= projectname;
this.genre= genre;
}
//Class collection
classCollection(){
return projectsCollection.push(this);
}
};
Seems so simple... yet it doesn't work! Any ideas to fix or alternatives to access all instances of a Class? Thank you Robin
2 Answers

Blake Larson
13,014 PointsThere are a couple ways you can do this. You just have to call that method on each instance of a new project.
const projectsCollection = [];
let Project = class {
constructor(projectname, genre){
this.projectname= projectname;
this.genre= genre;
}
//Class collection
classCollection(){
projectsCollection.push(this);
}
};
let p1 = new Project('song', 'country');
p1.classCollection();
let p2 = new Project('movie', 'comedy');
p2.classCollection();
console.log(projectsCollection); // Expects [Project, Project];
Or
const projectsCollection = [];
let Project = class {
constructor(projectname, genre){
this.projectname= projectname;
this.genre= genre;
}
};
let p1 = new Project('song', 'country');
projectsCollection.push(p1);
let p2 = new Project('movie', 'comedy');
projectsCollection.push(p2);
console.log(projectsCollection); // Expects [Project, Project];
Automatically on creation called in constructor
let projectsCollection = [];
let Project = class {
constructor(project, genre) {
this.project = project;
this.genre = genre;
this.pushProject = function() {
projectsCollection.push(this);
}
this.pushProject();
}
}
let p1 = new Project('song', 'country');
let p2 = new Project('movie', 'comedy');
console.log(projectsCollection); // Expects [Project, Project];

robinhickson
Front End Web Development Techdegree Graduate 18,162 PointsAhh. I like the last solution - the other two I had (finally) got working already. But the last solution is neat and tidy. Thank you!
Robin