Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial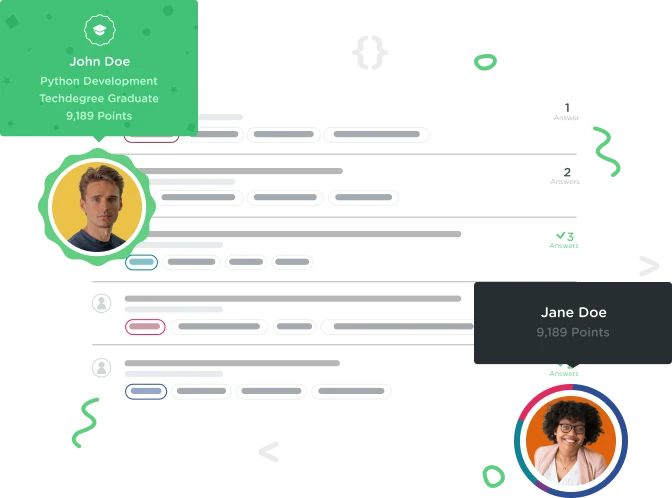

Herri Fransisca
922 Pointscan i just send you the code that i written in my visual studio to complete my final task of c# basics ? Thanks :)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
int numberOfHits = 0;
bool keepGoing = true;
while (keepGoing)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
if (entry == null)
{
Console.WriteLine("cannot empty");
continue;
}
else
{
if (entry == "quit")
{
keepGoing = false;
}
else
{
try
{
numberOfHits = int.Parse(entry);
}
catch (FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
if (numberOfHits < 0)
{
Console.WriteLine("Must be positive");
continue;
}
else
{
for (int x = 0; x < numberOfHits; x++)
{
Console.WriteLine("Yay!");
}
}
}
}
}
}
}
}
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
}
}
}
(Edited by BothXP to add markdown formatting.)
2 Answers
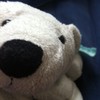
bothxp
16,510 PointsHi Herri,
The tests run against your code to make sure that you pass are automated. In this case the tests are not going to provide 'quit' as an answer so it's going to get stuck in your while loop. So I added a 'break' after the for loop had ran. Basically it is not expecting a while loop, so it's best not to add one.
Make sure you pay attention to the exact wording of the console.writeline statements that you have been asked to use. For example you had used "That is not valid input.", when to pass you needed to have used "You must enter a whole number.".
Modifying your code as little as I could, this passed all of the tasks:
using System;
namespace Treehouse.CodeChallenges {
class Program {
static void Main() {
int numberOfHits = 0;
bool keepGoing = true;
while (keepGoing) {
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
if (entry == null) {
Console.WriteLine("cannot empty");
continue;
} else {
if (entry == "quit") {
keepGoing = false;
} else {
try {
numberOfHits = int.Parse(entry);
}
catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
break;
}
if (numberOfHits < 0) {
Console.WriteLine("You must enter a positive number.");
break;
} else {
for (int x = 0; x < numberOfHits; x++) {
Console.WriteLine("Yay!");
}
break;
}
}
}
}
}
}
}
but to actually pass all 3 of the tasks you only need something like:
using System;
namespace Treehouse.CodeChallenges {
class Program {
static void Main() {
int numberOfHits = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
try {
numberOfHits = int.Parse(entry);
if (numberOfHits < 0) {
Console.WriteLine("You must enter a positive number.");
} else {
for (int x = 0; x < numberOfHits; x++) {
Console.WriteLine("Yay!");
}
}
}
catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
}
}
}
}
I hope that helps.

Herri Fransisca
922 PointsWould you mind giving me a Hint / Answer about my code below. why it couldn't finish the last task of c# basic ?
Thanks for the help ! :)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
int numberOfHits = 0;
bool keepGoing = true;
while (keepGoing)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
if (entry == null)
{
Console.WriteLine("cannot empty");
continue;
}
else
{
if (entry == "quit")
{
keepGoing = false;
}
else
{
try
{
numberOfHits = int.Parse(entry);
}
catch (FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
if (numberOfHits < 0)
{
Console.WriteLine("Must be positive");
continue;
}
else
{
for (int x = 0; x < numberOfHits; x++)
{
Console.WriteLine("Yay!");
}
}
}
}
}
}
}
}
(Edited by BothXP to add Markdown)