Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial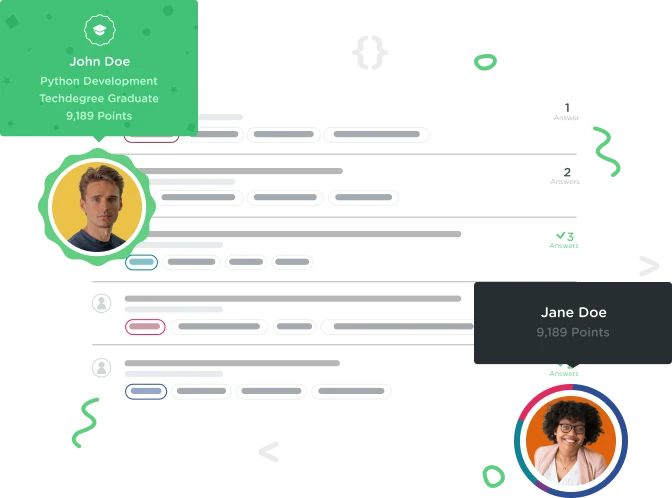

Travis John Villanueva
5,052 Pointscan somebody explain what does this mean? "Cannot implicitly convert type int to bool?" Thats what i have in my error
Im trying to compare the my current array value to my preceding one. I use a do while loop and inside that i have an conditional if loop that returns true if the value matches and false if it didn't.
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
int i=1;
int j = i-1;
while(i<10){
if(sequence[i]=sequence[j]){
return true;
}else{
return false;
}
}
}
}
}
1 Answer
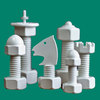
Steven Parker
241,956 PointsYou have a typo.
On line 11 of RepeatDetector.cs, you have an assignment (single "=") where you probably intended an equality comparison (double "==").
Also, when scanning for a match, you probably don't want that else clause because that will force a return before you have checked all the elements. Instead just return false after the loop is over. You should also compute your "j" value inside the loop, and the loop count should be based on the array size an not a fixed number.
Travis John Villanueva
5,052 PointsTravis John Villanueva
5,052 PointsHi Steven Parker,
Thank you for the guidance, and i want you to know that I gain knowledge on what you pointed out. I didn't just follow the direction, but understand the logic behind it.
I had done the:
however, struggling to code the consideration of array size, can you help me with this last challenge?
Steven Parker
241,956 PointsSteven Parker
241,956 PointsDid you remember to fix your loop count to be based on the array size instead of a fixed number?
If so, show the code as you have it now (remember proper formatting).