Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial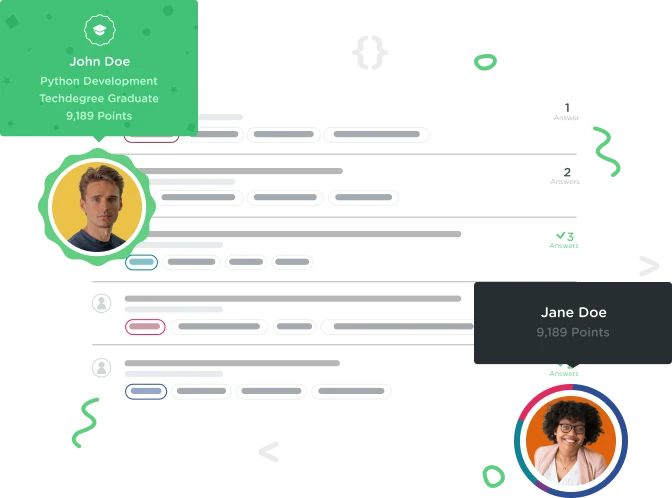
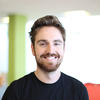
Kristian Woods
23,414 PointsCan someone clear up the PHP forloop in conjunction with associative arrays?
Hey, I'm retracing my steps in PHP because I'm rusty. My problem is with understanding associative arrays with the forloop.
for example:
<?php
$myArray = array("one", "two", "three");
foreach($myArray as $value) {
echo $value . "<br>";
}
?>
I know this loops through $myArray and assigns each element to $value
<?php
$myArray = array("one", "two", "three");
foreach($myArray as $key => $value) {
echo $key . " " . $value . "<br>";
}
?>
I also know that by including the $key variable, you now have access to the key/index associated with that element.
What I don't understand is this:
<?php
$myArray[0] = ["red" => "stop"];
$myArray[1] = ["green" => "go"];
foreach($myArray as $key => $value) {
echo $key . "<br>";
}
?>
My thinking was that the $key would be "red" and "green" - as they are the key to the values - "stop" and "go"
so, just to be clear - the first associative-array ["red" => "stop"], would be at index position 0 in $myArray and ["green" => "go"], would be at index 1? And in order to get the key in those internal arrays, would be to do another foreach loop inside the existing forloop?
<?php
$myarray[] = array ("blue" => "cold");
$myarray[] = array ("red" => "hot");
foreach($myarray as $key => $value) {
echo $key . $value . "<br>";
foreach($value as $innerkey => $innerValue) {
echo $innerkey . "=> " . $innerValue . "<br>";
}
}
?>
Thank You for any help
1 Answer

Darrell Conklin
22,099 PointsA secondary foreach loop is one way to handle iterating through multidimensional arrays. However depending on your circumstances there is always another way to accomplish what you want to do. You don't need to echo out the variable after instantiating it within the foreach loop, just pass it to the next loop like such.
<?php
$myArray[0] = ["red" => "stop"];
$myArray[1] = ["green" => "go"];
foreach ($myArray as $key => $value) {
foreach ($value as $innerKey => $innerValue) {
echo $innerKey . "<br>";
}
}
or you could do something like this
<?php
$myArray[0] = ["red" => "stop"];
$myArray[1] = ["green" => "go"];
foreach ($myArray as $key => $value) {
echo key($value) . '<br>';
}
Another way to get your inner values.
<?php
$myArray[0] = ["red" => "stop"];
$myArray[1] = ["green" => "go"];
for ($i = 0; $i < count($myArray); $i++) {
foreach ($myArray[$i] as $key => $value) {
echo $key . " = " . $value . "<br>";
}
}
If the keys in the inner arrays were the same you could do something like this:
<?php
$myArray[0] = [
"color" => "red",
"action" => "stop"
];
$myArray[1] = [
"color" => "green",
"action" => "go"
];
foreach ($myArray as $innerArray) {
echo $innerArray['color'] . " = ". $innerArray['action'] . "<br>";
}
but the main idea is that a foreach loop can only iterate over one array at a time. The $key variable holding the initial key and the $value holding the associated value of the key, in this case, another array. Getting the keys and values within the inner array can be done in many different ways.

Darrell Conklin
22,099 PointsSorry I know it's been 10 days but I was just searching through the forums for posts with 0 answers
Dmitriy Davydov
8,768 PointsDmitriy Davydov
8,768 PointsHey Kristian! Within the first (outer) loop you can't impress $value with echo() becase that is an array. I changed a little bit your code so it works now : <?php
$myarray[] = array ("blue" => "cold"); $myarray[] = array ("red" => "hot");
foreach($myarray as $key => $value) { echo $key . " : "; foreach($value as $innerkey => $innerValue) { echo $innerkey . "=> " . $innerValue . "<br>"; } }
?>