Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial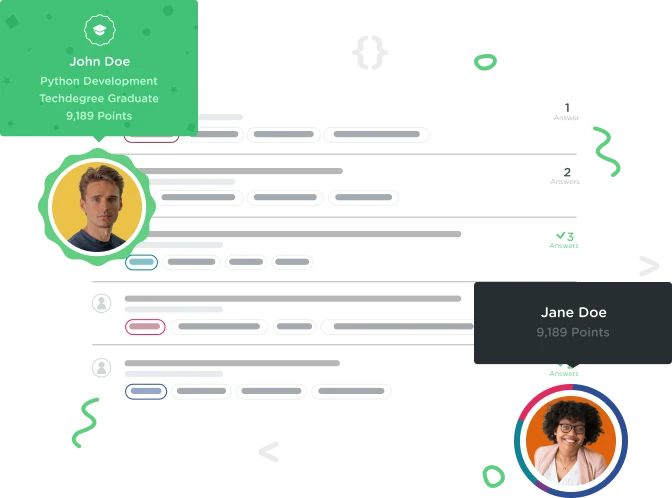

Abdurashid Mahad Isse
7,062 PointsCan someone help me with this challenge plz
4 Answers
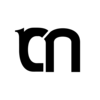
Colin Marshall
32,861 PointsI would be glad to help you with this.
Challenge Task 1 of 2
Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
First, we want to create a new function called max. Here is syntax for creating a new function. Note that this is not the only way to create a new function, I just went with the way they chose in the video.
function max() {
}
Next, the function needs to take two numbers as arguments. Arguments go inside the parentheses after the function name. Multiple arguments are separated by commas. Arguments are values that are passed into the function. Those values are then used inside of the function. When you write a function, you can think of the arguments as placeholders that are replaced by the values that you pass through the function when the function is called.
function max(num1, num2) {
}
Finally, it wants us to add a conditional statement, which returns the number with the higher value. Conditional statements are if statements. To decide what a function returns, you simply use the return
keyword.
function max(num1, num2) {
if (num1 > num2) {
return num1;
} else if (num1 < num2) {
return num2;
}
}
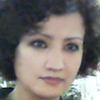
Durdona Abdusamikovna
1,553 PointsMy answer was slightly different but the same result :)
function max (large, small){
if (large > small) {
return large;
} else {
return small;
}
}
console.log(max(90, 11));
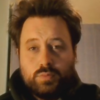
Andrew Shipley
7,781 PointsI was stuck on this too I got:
function max(num1, num2){
num1 = 1;
num2 = 2;
if (num1 > num2) {
return num1;
}
else{
return num2;
}
}
Can someone explain why its wrong please?
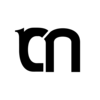
Colin Marshall
32,861 PointsYou shouldn't set the value of num1 and num2 inside the function. num1 and num2 are arguments that will be different every time you call the function. To run the function with with num1 equal to 1 and num2 equal to 2, you would call the function like this:
max(1, 2);
num1 and num2 can be any number. If I call the function with 5 and 3, and you have num1 and num2 set to be 1 and 2 inside of the function, it won't work properly because it will override the 5 and 3.
max(5, 3);
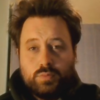
Andrew Shipley
7,781 PointsThanks everyone, sorry about the delayed reply, was taking a break :)